- Published on
Stacks: Mastering the LIFO Powerhouse (For Beginners and Beyond)
- Authors
-
-
- Name
- Jitendra M
- @_JitendraM
-
Table of contents:
-
Introduction
-
What is a Stack?
- How Does a Stack Work?
-
Time Complexity
-
Space Complexity
-
Pseudo Code
-
Stack in Python
-
Stack in C++
-
Comparison with Other Data Structures
-
Stack vs. Queue: Choosing the Right Tool
-
Stacks in Action: Beyond Theory
-
Pros and Cons
-
Real-World Applications
-
Conclusion
-
Explore More Topics
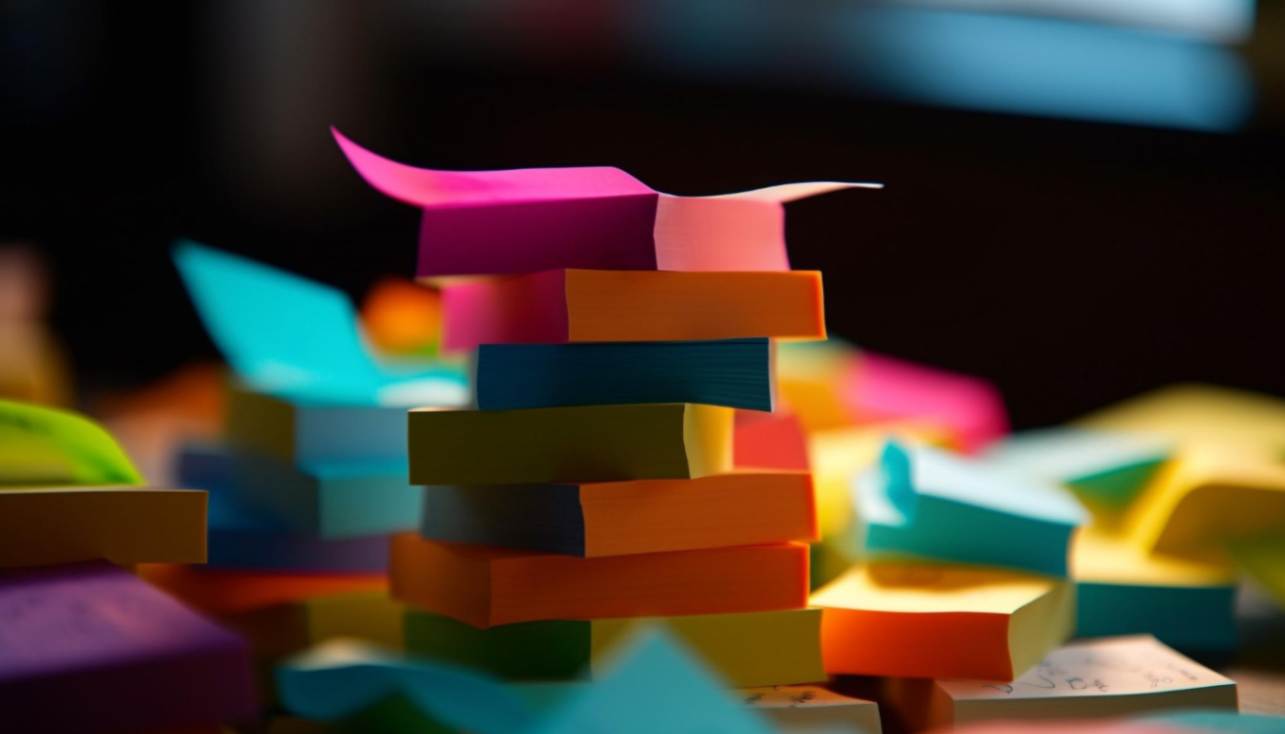
Introduction
In the realm of computer science and data structures, stacks play a pivotal role. A stack is a dynamic data structure that follows the Last In, First Out (LIFO) principle. In simpler terms, the last element added is the first one to be removed. Understanding the basics of stacks is crucial for tackling a variety of computational challenges.
What is a Stack?
A stack is a collection of elements with two primary operations: push, which adds an element to the collection, and pop, which removes the last-added element. Consider it as a metaphorical stack of plates in a cafeteria – the last plate placed is the first one taken off.
How Does a Stack Work?
Basic Operations:
- Push: Adding an element to the stack.
- Pop: Removing the most recently added element.
- Peek: Viewing the top element without removal.
Visualization:
Imagine stacking books; the last book placed becomes the base, and the first one removed is from the top. This visualizes the LIFO behavior inherent in a stack.
Time Complexity
The fundamental operations of a stack – push, pop, and peek – generally have a constant time complexity of O(1)
. This efficiency makes stacks suitable for various applications.
Space Complexity
Stacks are also memory-friendly. Their space complexity is also O(1)
, meaning they use a fixed amount of memory per operation, regardless of the stack’s size. This makes them lightweight and efficient even for large datasets.
Pseudo Code
procedure push(stack, element):
stack.push(element)
procedure pop(stack):
if stack is not empty:
stack.pop()
procedure peek(stack):
if stack is not empty:
return stack.top()
Stack in Python
class Stack:
def __init__(self):
self.items = []
def push(self, item):
self.items.append(item)
def pop(self):
if not self.is_empty():
return self.items.pop()
def peek(self):
if not self.is_empty():
return self.items[-1]
def is_empty(self):
return len(self.items) == 0
Stack in C++
#include <iostream>
#include <stack>
int main() {
std::stack<int> mystack;
mystack.push(1);
mystack.push(2);
mystack.push(3);
while (!mystack.empty()) {
std::cout << mystack.top() << " ";
mystack.pop();
}
return 0;
}
Comparison with Other Data Structures
In contrast to stacks, queues follow the First In, First Out (FIFO) principle. While stacks excel in managing function calls and undo operations, queues are apt for scenarios such as print job scheduling.
Stack vs. Queue: Choosing the Right Tool
While stacks excel at managing function calls and undo operations, their LIFO nature differs from queues, which follow First In, First Out (FIFO). Think of a queue like a line at the bakery; the first person in line gets served first. This makes queues ideal for scenarios like print job scheduling or processing tasks in order.
Stacks in Action: Beyond Theory
Stacks power various real-world applications:
- Function Calls: Stacks manage the order of function calls in a program, ensuring proper execution and memory allocation.
- Expression Parsing: Stacks help evaluate complex mathematical expressions by processing operators and operands in the correct order.
- Undo Mechanism: Stacks enable undo functionality in applications, allowing you to rewind and retrace your steps.
- Balancing Parentheses: Stacks verify the correct placement of opening and closing parentheses in code or mathematical expressions.
- Browser History: Stacks keep track of your browsing history, allowing you to navigate back and forth between visited pages.
- Backtracking Algorithms: Stacks play a crucial role in backtracking algorithms like mazesolver and graph traversal.
Pros and Cons
Pros:
- Efficient and constant time complexity.
- Ideal for managing function calls and undo operations.
Cons:
- Limited in functionality compared to more complex data structures.
Real-World Applications
- Function Calls: Managing function calls in a program.
- Expression Parsing: Evaluating mathematical expressions.
- Undo Mechanism: Enabling the undo functionality in applications.
Conclusion
Stacks, with their simplicity and efficiency, hold a significant place in computer science. Mastering the fundamentals of stacks provides a powerful tool for solving diverse computational problems. As you delve deeper into the world of data structures, understanding when and how to use stacks becomes a valuable skill.