- Published on
Understanding AVL Trees: A Self-Balancing Binary Search Tree
- Authors
-
-
- Name
- Jitendra M
- @_JitendraM
-
Table of contents:
-
Introduction
-
What is an AVL Tree?
-
Balancing Operations
- Operations in an AVL Tree
-
Properties of AVL Trees
-
Time Complexity
-
Example
-
AVL Tree in Python
-
AVL Tree in C++
- Pros and Cons
-
Advanced AVL Tree Topics
-
Choosing the Right Data Structure
-
Comparison with Other Tree Algorithms
-
Real-World Applications
-
Conclusion
-
Explore more topics
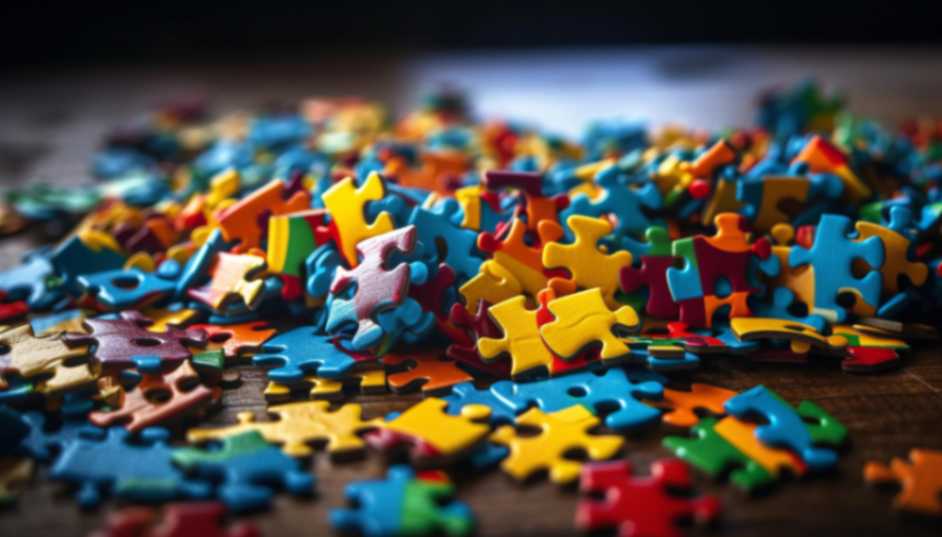
Introduction
AVL trees are a type of self-balancing binary search tree (BST) named after their inventors, Adelson-Velsky and Landis. They maintain balance in the tree by performing rotations when necessary during insertion and deletion operations. This self-balancing property ensures that the height of the tree remains logarithmic, which results in efficient search, insertion, and deletion operations.
What is an AVL Tree?
An AVL tree is a binary search tree with the additional property that the heights of the two child subtrees of every node differ by at most one. This property is known as the “balance factor.” The balance factor of a node is calculated as the height of its right subtree minus the height of its left subtree.
Balancing Operations
To maintain the balance factor and keep the tree balanced, AVL trees perform rotations during insertion and deletion operations. The four types of rotations are:
- Left Rotation
- Right Rotation
- Left-Right Rotation (Double Right Rotation)
- Right-Left Rotation (Double Left Rotation)
These rotations help ensure that the balance factor of each node remains within the acceptable range, typically -1
, 0
, or 1
.
Operations in an AVL Tree
Insertion
When inserting a new node into an AVL tree:
- Compare the value with the current node.
- If it’s less than the current node, move to the left subtree; if greater, move to the right.
- Repeat this process until you find an empty spot (a leaf node) to insert the new value.
Deletion
Deleting a node in an AVL tree requires careful handling to maintain the AVL property:
- If the node to delete is a leaf or has only one child, simply remove it.
- If it has two children, find the in-order predecessor or successor (the node with the closest value), replace the node to delete with it, and then delete the predecessor or successor.
Search
Searching in an AVL tree is performed like a regular binary search tree. Thanks to its self-balancing property, the average time complexity for searching in an AVL tree is O(log n).
Properties of AVL Trees
-
AVL trees maintain their balance during insertions and deletions, ensuring that the height remains logarithmic, resulting in efficient operations.
-
The height of an AVL tree with ‘n’ nodes is
O(log n)
. -
The balance factor of every node is between
-1
,0
, or1
, indicating the balance of the tree.
Time Complexity
The time complexity for search, insert, and delete operations in an AVL tree is O(log n)
, where ‘n’ is the number of nodes.
Balancing operations (rotations) take constant time.
Example
Consider the following AVL tree:
30
/ \
20 40
/ \ \
10 25 50`
Let’s insert the value 5 into the tree:
30
/ \
10 40
/ \ \
5 20 50
\
25
Now, the tree is unbalanced. We can perform rotations to balance it:
30
/ \
10 40
/ \ \
5 20 50
\
25
AVL Tree in Python
class AVLNode:
def __init__(self, key):
self.key = key
self.left = None
self.right = None
self.height = 1
class AVLTree:
def insert(self, root, key):
# Standard BST insertion
if not root:
return AVLNode(key)
if key < root.key:
root.left = self.insert(root.left, key)
else:
root.right = self.insert(root.right, key)
# Update height of the current node
root.height = 1 + max(self.get_height(root.left), self.get_height(root.right))
# Perform rotations to balance the tree
return self.balance(root)
AVL Tree in C++
struct AVLNode {
int key;
AVLNode* left;
AVLNode* right;
int height;
};
class AVLTree {
public:
AVLNode* insert(AVLNode* root, int key) {
// Standard BST insertion
if (!root) {
return new AVLNode{key, nullptr, nullptr, 1};
}
if (key < root->key) {
root->left = insert(root->left, key);
} else {
root->right = insert(root->right, key);
}
// Update height of the current node
root->height = 1 + std::max(getHeight(root->left), getHeight(root->right));
// Perform rotations to balance the tree
return balance(root);
}
};
Pros and Cons
Pros:
-
Efficient search, insert, and delete operations with a time complexity of
O(log n)
. -
Automatic self-balancing ensures the tree’s height remains logarithmic.
Cons:
- Slightly more complex to implement compared to regular binary search trees.
Advanced AVL Tree Topics
Advanced topics related to AVL trees include:
-
Self-Balancing Variants: Variations of AVL trees, such as Red-Black trees and Splay trees, provide alternative approaches to self-balancing.
-
Deletion Algorithms: In-depth exploration of AVL tree deletion algorithms.
Choosing the Right Data Structure
When selecting a data structure, consider the specific requirements of your application. AVL trees are well-suited for scenarios where frequent insertions, deletions, and searches are required while maintaining a balanced structure.
Comparison with Other Tree Algorithms
While AVL trees are powerful, other tree algorithms like B-trees and Red-Black trees also have their strengths and use cases. Comparing these algorithms can help you make an informed choice for your application.
Real-World Applications
AVL trees find applications in various fields:
-
Database Management Systems: Used for indexing and efficient data retrieval.
-
File Systems: Some file systems use AVL trees for efficient file indexing and retrieval.
-
Networking: AVL trees play a role in IP routing tables for quick lookups.
-
Language Compilers: Used in compilers for symbol tables and expression parsing.
Conclusion
AVL trees are fundamental data structures that provide efficient search, insertion, and deletion operations. Their self-balancing property ensures that the tree remains balanced even during dynamic operations. Understanding AVL trees and their applications is valuable in computer science and real-world scenarios.
Now that you’ve learned about AVL trees, consider exploring more advanced topics, self-balancing variants, and comparisons with other tree-based data structures to expand your knowledge.