- Published on
Fenwick Trees: Efficient Data Structure for Range Queries
- Authors
-
-
- Name
- Jitendra M
- @_JitendraM
-
Table of contents:
-
Introduction
-
What is a Fenwick Tree?
- How Fenwick Trees Work
-
Time and Space Complexity
-
Pseudo Code for Fenwick Trees
-
Fenwick Tree in Python
-
Fenwick Tree in C++
-
Practical Applications
-
Comparison with Other Data Structures
- Pros and Cons
-
Conclusion
-
Explore More
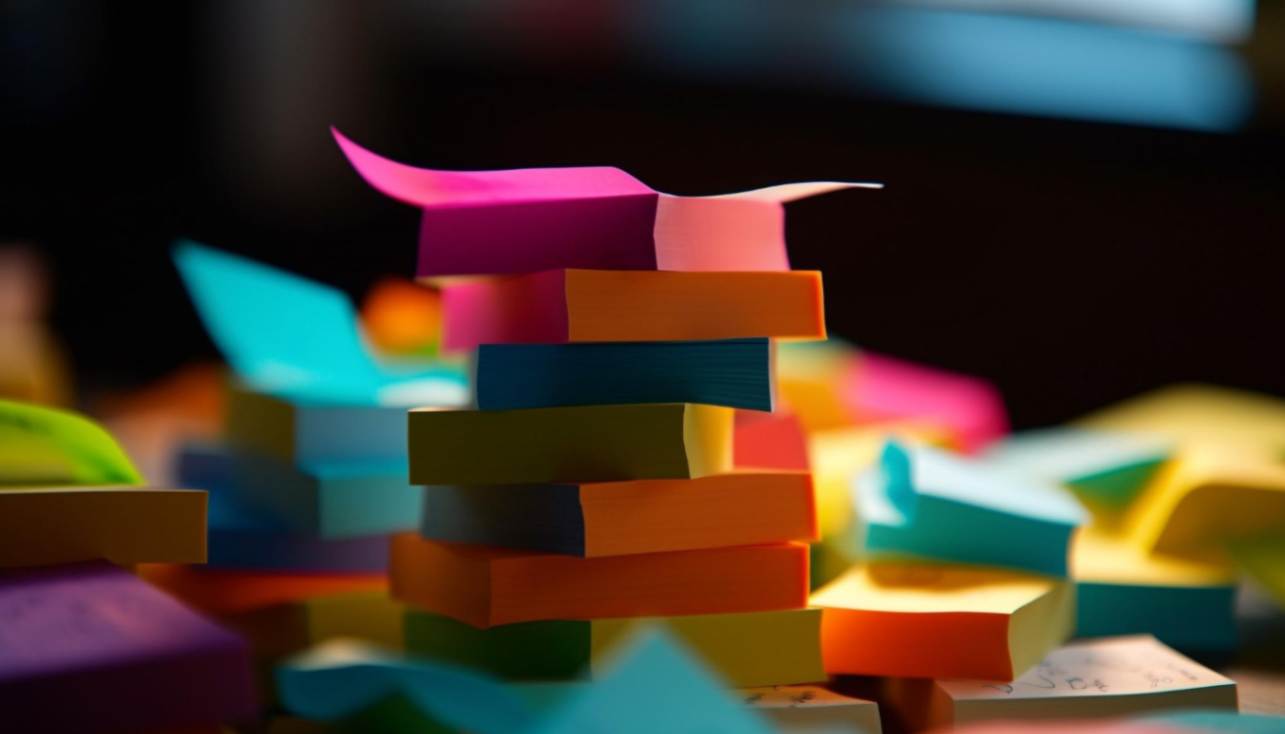
Introduction
Fenwick Trees, also known as Binary Indexed Trees (BITs), are a clever and efficient data structure used for handling range queries in arrays. They’re particularly handy for tasks like cumulative frequency calculations, point updates, and more. In this article, we’ll delve into what Fenwick Trees are, how they work, their time complexity, practical applications, and even provide a Python implementation.
What is a Fenwick Tree?
A Fenwick Tree is a binary tree-like data structure used to maintain the cumulative sum of an array of values. It’s a compact and elegant solution for handling various range queries efficiently.
How Fenwick Trees Work
Fenwick Trees use a cunning approach to compute cumulative frequencies. The key idea is to represent an array element at a specific index by including it in the cumulative frequency of other elements. It allows for efficient updates and queries.
Insertion
Adding a value to a Fenwick Tree involves traversing the tree to find specific nodes to update while maintaining the structure’s integrity. This is the foundation for various range query operations.
Querying
Querying in a Fenwick Tree is equally clever. It computes the cumulative sum for a range by carefully selecting a few nodes in the tree.
Time and Space Complexity
- Time Complexity:
- Update Operation:
O(log n)
- Query Operation:
O(log n)
- Update Operation:
- Space Complexity:
O(n)
Pseudo Code for Fenwick Trees
Here’s a simple pseudo code representation of a Fenwick Tree update operation:
function update(tree, index, value):
while index <= size:
tree[index] += value
index += index & -index
Fenwick Tree in Python
Let’s take a closer look at how to implement a Fenwick Tree in Python.
class FenwickTree:
def __init__(self, n):
self.size = n
self.tree = [0] * (n + 1)
def update(self, index, value):
while index <= self.size:
self.tree[index] += value
index += index & -index
def query(self, index):
total = 0
while index:
total += self.tree[index]
index -= index & -index
return total
Fenwick Tree in C++
In addition to the Python implementation, here’s a simple C++ implementation of a Fenwick Tree:
#include <vector>
class FenwickTree {
public:
FenwickTree(int n) : size(n), tree(n + 1) {}
void update(int index, int value) {
while (index <= size) {
tree[index] += value;
index += index & -index;
}
}
int query(int index) {
int total = 0;
while index {
total += tree[index];
index -= index & -index;
}
return total;
}
private:
int size;
std::vector<int> tree;
};
Practical Applications
Fenwick Trees find applications in various areas, including:
- Computational Geometry: They can efficiently handle geometric queries like counting points within a certain range.
- Frequency Counting: Fenwick Trees are excellent for counting the frequency of elements in a range.
- Dynamic Programming: They’re widely used in solving dynamic programming problems that involve range queries.
- Summarization Queries: When dealing with large datasets, Fenwick Trees help speed up operations like summarization queries.
Comparison with Other Data Structures
Fenwick Trees are tailored for efficient cumulative frequency calculations and are specialized for this purpose. When you need to perform such operations, they outperform general-purpose data structures like arrays or linked lists.
Pros and Cons
Pros:
- Efficient for range queries
- Low memory footprint
- Easy to implement
Cons:
- Limited to specific operations
- May require additional data structures for complex queries
Conclusion
Fenwick Trees, or Binary Indexed Trees, offer an elegant solution to a common problem in computer science and data processing. They’re invaluable when it comes to managing range queries, and their efficient time complexity makes them a go-to choice for many applications. With the Python and C++ implementations provided, you’re now equipped to leverage the power of Fenwick Trees in your projects.
Explore More
To expand your knowledge in data structures, consider exploring other advanced topics like Segment Trees, Hashing, or advanced tree structures. These versatile tools can be instrumental in solving various computational challenges.