- Published on
Simplify Laravel Development: A Guide to BelongsToMany Relationships
- Authors
-
-
- Name
- Jitendra M
- @_JitendraM
-
Table of contents:
-
Introduction
- What is a BelongsToMany Relationship? πππ€
-
Why Should Developers Care About Many-to-Many Relationships? π§
- Setting Up a Many-to-Many Relationship π οΈππ»
- Example: Students and Courses ππ«π¨βπ
- Adding Additional Data to the Pivot Table π ππ
- Using attach, sync, and detach π οΈππ€
-
Comparison with Other Relationship Types π€ππ‘
-
Best Practices and Tips πππ
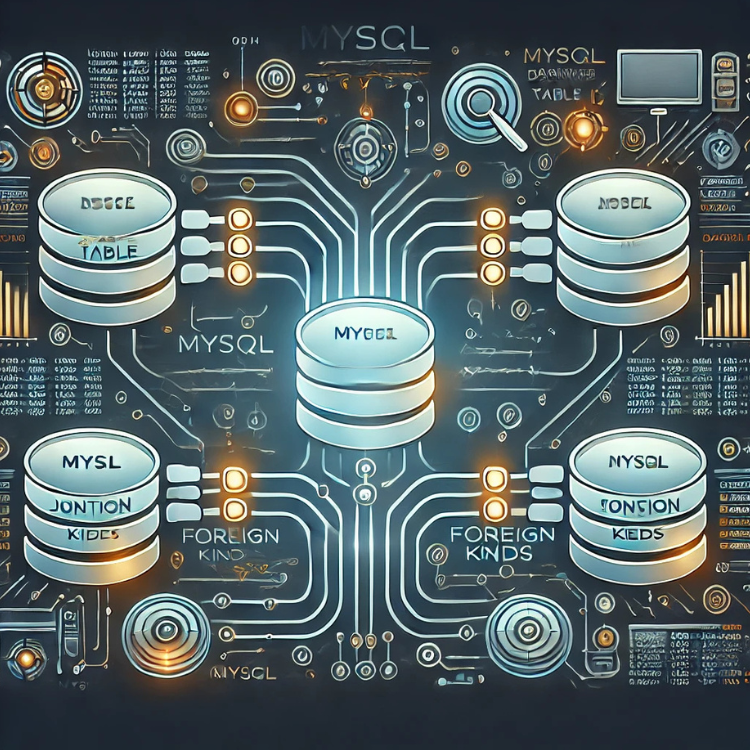
Introduction
The BelongsToMany
relationship in Laravel is a powerful feature that simplifies handling many-to-many relationships in your database. This article will guide you through understanding and implementing the BelongsToMany
relationship using simple language, trending concepts, and practical examples.
What is a BelongsToMany
Relationship? πππ€
Imagine this scenario:
- A student can enroll in multiple courses. π
- A course can have multiple students. π
This is what we call a many-to-many relationship in the database world. Unlike one-to-many relationships (where each record in one table is related to only one or more records in another table), many-to-many relationships require a middle table (often called a pivot table) to connect the two.
Think of it like this:
-
Students are connected to Courses through an enrollment process.
-
The pivot table acts like a guest list at a party β it tells you which students are enrolled in which courses.
Hereβs how it looks in database terms:
-
students table: Holds the details about students (e.g.,
id
,name
). -
courses table: Holds the details about courses (e.g.,
id
,title
). -
students_courses table: Links students and courses together (e.g.,
student_id
,course_id
).
Real-Life Examples of Many-to-Many Relationships
-
Tags and Articles: An article can have multiple tags, and a tag can belong to multiple articles. π·οΈ
-
Authors and Books: An author can write multiple books, and a book can have multiple authors. π
-
Movies and Actors: A movie can feature multiple actors, and an actor can work in multiple movies. π₯
Why Should Developers Care About Many-to-Many Relationships? π§
If youβve ever struggled with deciding how to represent complex relationships in your application, understanding many-to-many is your lightbulb moment. π‘ Itβs the foundation for building scalable and flexible data models.
Setting Up a Many-to-Many Relationship π οΈππ»
To define a BelongsToMany
relationship, follow these steps:
Step 1: Create Models and Migrations
Run the following Artisan commands to create models and migrations:
php artisan make:model Student -m
php artisan make:model Course -m
php artisan make:migration create_course_student_table
Step 2: Define Pivot Table Schema
In the create_course_student_table
migration, define the table structure:
Schema::create('course_student', function (Blueprint $table) {
$table->id();
$table->unsignedBigInteger('student_id');
$table->unsignedBigInteger('course_id');
$table->timestamps();
$table->foreign('student_id')->references('id')->on('students')->onDelete('cascade');
$table->foreign('course_id')->references('id')->on('courses')->onDelete('cascade');
});
Step 3: Define Relationships in Models
To create relationships in Laravel, you also need to understand the role of foreign keys. These keys act as a bridge connecting two tables in a database, ensuring that related data stays consistent. In many-to-many relationships, the foreign keys in the pivot table link the primary keys of the related tables.
For example, if you have a course_student
pivot table, it will include student_id
and course_id
as foreign keys. Hereβs how you define them in the migration file:
Schema::create('course_student', function (Blueprint $table) {
$table->id();
$table->unsignedBigInteger('student_id');
$table->unsignedBigInteger('course_id');
$table->timestamps();
$table->foreign('student_id')->references('id')->on('students')->onDelete('cascade');
$table->foreign('course_id')->references('id')->on('courses')->onDelete('cascade');
});
Foreign keys ensure that you cannot add a record to the course_student
table unless the referenced student and course exist in their respective tables. This integrity is crucial for maintaining accurate relationships in your database.
In the Student
model:
public function courses() {
return $this->belongsToMany(Course::class);
}
In the Course
model:
public function students() {
return $this->belongsToMany(Student::class);
}
Example: Students and Courses ππ«π¨βπ
Letβs say we want to enroll a student in multiple courses:
Attaching Relationships
$student = Student::find(1);
$student->courses()->attach([1, 2, 3]);
This will insert rows in the course_student
pivot table linking the student with IDs 1
, 2
, and 3
to the courses with these IDs.
Fetching Relationships
$student = Student::find(1);
$courses = $student->courses;
foreach ($courses as $course) {
echo $course->name;
}
Adding Additional Data to the Pivot Table π ππ
Sometimes, you may want to store additional information in the pivot table, such as enrollment date or grade.
Modify Pivot Table Schema
Update the migration to include additional fields:
Schema::create('course_student', function (Blueprint $table) {
$table->id();
$table->unsignedBigInteger('student_id');
$table->unsignedBigInteger('course_id');
$table->date('enrolled_at');
$table->string('grade')->nullable();
$table->timestamps();
});
Use withPivot
In the Student
model:
public function courses() {
return $this->belongsToMany(Course::class)->withPivot('enrolled_at', 'grade');
}
Insert Data with Pivot Fields
$student->courses()->attach([1 => ['enrolled_at' => now(), 'grade' => 'A']]);
Retrieve Pivot Data
$courses = $student->courses;
foreach ($courses as $course) {
echo $course->pivot->enrolled_at;
echo $course->pivot->grade;
}
Using attach
, sync
, and detach
π οΈππ€
Laravel offers three key methods for managing records in a pivot table: attach
, sync
, and detach
. Each of these serves a specific purpose:
-
attach
: Adds new relationships without altering existing ones. -
sync
: Updates the relationships to match the provided array, removing any that are not included. -
detach
: Removes specified relationships.
Letβs dive deeper into these methods with examples to understand their usage better:
attach
The attach
method adds new relationships. For example:
$student->courses()->attach([1, 2]);
This command inserts new entries in the pivot table linking the student to the courses with IDs 1
and 2
.
sync
The sync
method updates the pivot table to match the provided array, removing any relationships not included:
$student->courses()->sync([1, 3]);
Here, the pivot table will only retain the relationships with course IDs 1
and 3
. Any other existing relationships will be removed.
detach
The detach
method removes specific relationships. For instance:
$student->courses()->detach([2]);
This command deletes the pivot table entry that links the student to the course with ID 2
.
By using these methods effectively, you can easily manage your many-to-many relationships in Laravel.
Comparison with Other Relationship Types π€ππ‘
Feature | BelongsToMany |
HasMany |
HasOne |
---|---|---|---|
Pivot Table Support | Yes | No | No |
Many-to-Many Relation | Yes | No | No |
Use Case | Students & Courses | Posts & Comments | User & Profile |
Best Practices and Tips πππ
-
Name Pivot Tables Properly: Use alphabetical order, e.g.,
course_student
instead ofstudent_course
. -
Eager Loading: Use
with()
to optimize queries and avoid N+1 problems.$students = Student::with('courses')->get();
-
Validate Input: Ensure IDs passed to
attach
orsync
exist in the related table to avoid errors. -
Use
syncWithoutDetaching
: To add relationships without removing existing ones:$student->courses()->syncWithoutDetaching([4]);
By following these guidelines and examples, you can effectively manage many-to-many relationships in Laravel. Mastering BelongsToMany
can greatly simplify your database operations and improve your applicationβs performance and maintainability.