- Published on
Unlocking Laravel's Power: A Deep Dive into HasMany Relationships
- Authors
-
-
- Name
- Jitendra M
- @_JitendraM
-
Table of contents:
-
๐ Introduction
-
๐ค Understanding HasMany Relationships
- ๐ง Setting Up the Relationship
-
๐ Defining the Relationship in Models
-
๐ Querying HasMany Relationships
- ๐ Managing Relationships
-
๐ Eager Loading for Performance
-
๐ Best Practices and Tips
- ๐จ Avoiding Common Errors
-
Conclusion ๐
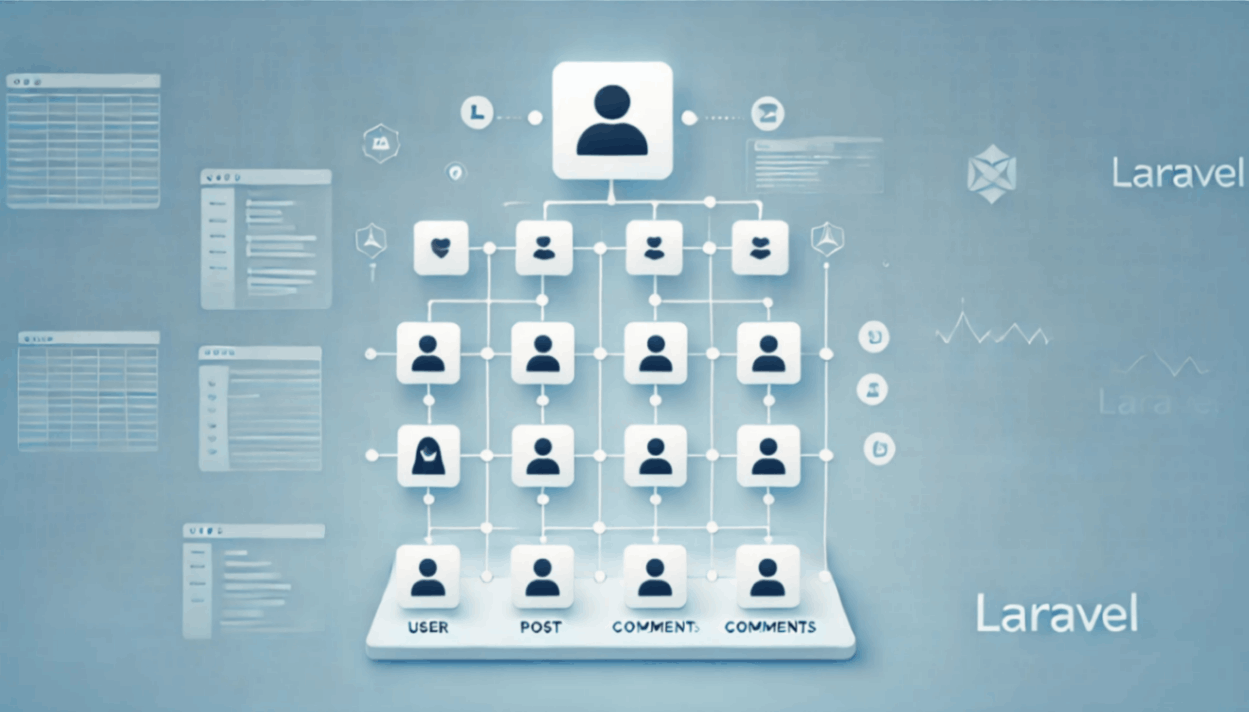
๐ Introduction
When building a robust Laravel application, defining and managing relationships between entities is crucial. The HasMany
relationship is one of the most commonly used relationships in Laravel. It represents a one-to-many connection, such as a user having multiple posts or a category containing several products.
In this guide, weโll simplify the concept and show you how to implement HasMany
relationships in Laravel step by step. ๐
๐ค Understanding HasMany
Relationships
Have you ever wondered how to model scenarios like:
- A blog post having multiple comments? ๐
- An online store category containing several products? ๐
- A user owning multiple posts? ๐ค
The HasMany
relationship handles these cases beautifully. It ensures your database reflects these associations accurately.
๐ง Setting Up the Relationship
1๏ธโฃ Create Models and Migrations
Letโs start by creating the necessary models and migrations. For instance, to set up a User
model and a Post
model:
php artisan make:model User -m
php artisan make:model Post -m
Question: Can you think of a real-world scenario in your project where one entity owns many others?
2๏ธโฃ Add Foreign Key in the Related Table
In the posts
table migration, define a foreign key referencing the users
table:
Schema::create('posts', function (Blueprint $table) {
$table->id();
$table->unsignedBigInteger('user_id');
$table->string('title');
$table->text('body');
$table->timestamps();
$table->foreign('user_id')->references('id')->on('users')->onDelete('cascade');
});
๐ Defining the Relationship in Models
Once the database is ready, define the relationship in the User
model:
public function posts()
{
return $this->hasMany(Post::class);
}
And in the Post
model, define the inverse relationship:
public function user()
{
return $this->belongsTo(User::class);
}
Question: How does defining relationships in models make your code cleaner and easier to understand? ๐ก
๐ Querying HasMany
Relationships
To retrieve all posts by a user:
$user = User::find(1);
$posts = $user->posts;
Need to filter? Try this:
$recentPosts = $user->posts()->where('created_at', '>', now()->subMonth())->get();
Tip: Think about optimizing your queries by retrieving only the data you need. โก
๐ Managing Relationships
โ Adding Related Records
Add a new post for a user:
$user->posts()->create([
'title' => 'My First Post',
'body' => 'This is the content of the post.',
]);
โ๏ธ Updating Related Records
Update a specific post:
$post = $user->posts()->first();
$post->update(['title' => 'Updated Title']);
๐๏ธ Deleting Related Records
Delete all posts of a user:
$user->posts()->delete();
Question: How would you handle deleting related records without accidentally removing too much data?
๐ Eager Loading for Performance
Fetching related records efficiently is crucial. Use eager loading to optimize queries:
$users = User::with('posts')->get();
This retrieves users along with their posts in a single query.
๐ Best Practices and Tips
-
๐ Database Indexing: Ensure the foreign key column (
user_id
) in theposts
table is indexed for faster queries. - ๐งน Clean Code: Offload complex queries to the model or service classes instead of placing them in controllers.
- ๐ Consistent Naming: Follow Laravelโs conventions for table and column names for better readability and maintainability.
Hereโs the updated article with an additional heading on avoiding common errors:
๐จ Avoiding Common Errors
Even seasoned Laravel developers can run into pitfalls when working with HasMany
relationships. Here are some common mistakes and how to avoid them:
1๏ธโฃ Forgetting to Set the Foreign Key
If the foreign key is not defined correctly in the migration, Laravel wonโt know how to link the two models.
Fix: Always add the foreign key in the migration using unsignedBigInteger
and the foreign()
method:
$table->unsignedBigInteger('user_id');
$table->foreign('user_id')->references('id')->on('users')->onDelete('cascade');
2๏ธโฃ Not Using the Correct Relationship Method
Sometimes developers mistakenly use belongsTo
instead of hasMany
in the parent model.
Fix: Double-check that the parent model uses hasMany
, while the child model uses belongsTo
.
3๏ธโฃ Missing Eager Loading
Retrieving related records without eager loading can lead to the N+1 query problem, causing performance issues.
Fix: Use eager loading with the with()
method when fetching models:
$users = User::with('posts')->get();
4๏ธโฃ Incorrect Naming Conventions
Laravel relies on conventions for foreign key and table names. Not following these can break your relationship setup.
Fix: Use standard naming conventions:
- Foreign key:
<singular_model_name>_id
(e.g.,user_id
) - Table names: plural and snake_case (e.g.,
users
,posts
).
5๏ธโฃ Not Handling Cascading Deletes Properly
When a parent model is deleted, related records might be left orphaned if cascading deletes are not configured.
Fix: Use onDelete('cascade')
in your foreign key definition to ensure related records are removed:
$table->foreign('user_id')->references('id')->on('users')->onDelete('cascade');
6๏ธโฃ Overcomplicating Queries
Some developers overuse raw SQL or complex logic in controllers for fetching related records.
Fix: Let Laravelโs Eloquent ORM handle the relationships and queries for you. Keep your controllers clean by leveraging model methods or service classes.
Question: Have you encountered any of these mistakes in your Laravel journey? How did you resolve them? ๐ ๏ธ
Conclusion ๐
By mastering the HasMany relationship, you can create more robust and efficient Laravel applications. This foundation will empower you to build complex data models and handle intricate relationships with ease.
By following this guide, youโll master the HasMany
relationship in Laravel and be well on your way to building scalable, efficient applications. ๐ช Relationships are the backbone of any relational database-driven application, and understanding them is crucial to becoming a Laravel pro. ๐