- Published on
Mastering Binary Search: A Comprehensive Guide for Efficient Searching
- Authors
-
-
- Name
- Jitendra M
- @_JitendraM
-
Table of contents:
-
Introduction
-
What is Binary Search?
-
Visual Representation
-
The Binary Search Process
-
Binary Search Flowchart
- Let’s Code!
- Section 4: Pros and Cons
-
Section 5: Real-World Examples
-
Conclusion
-
Explore More Topics
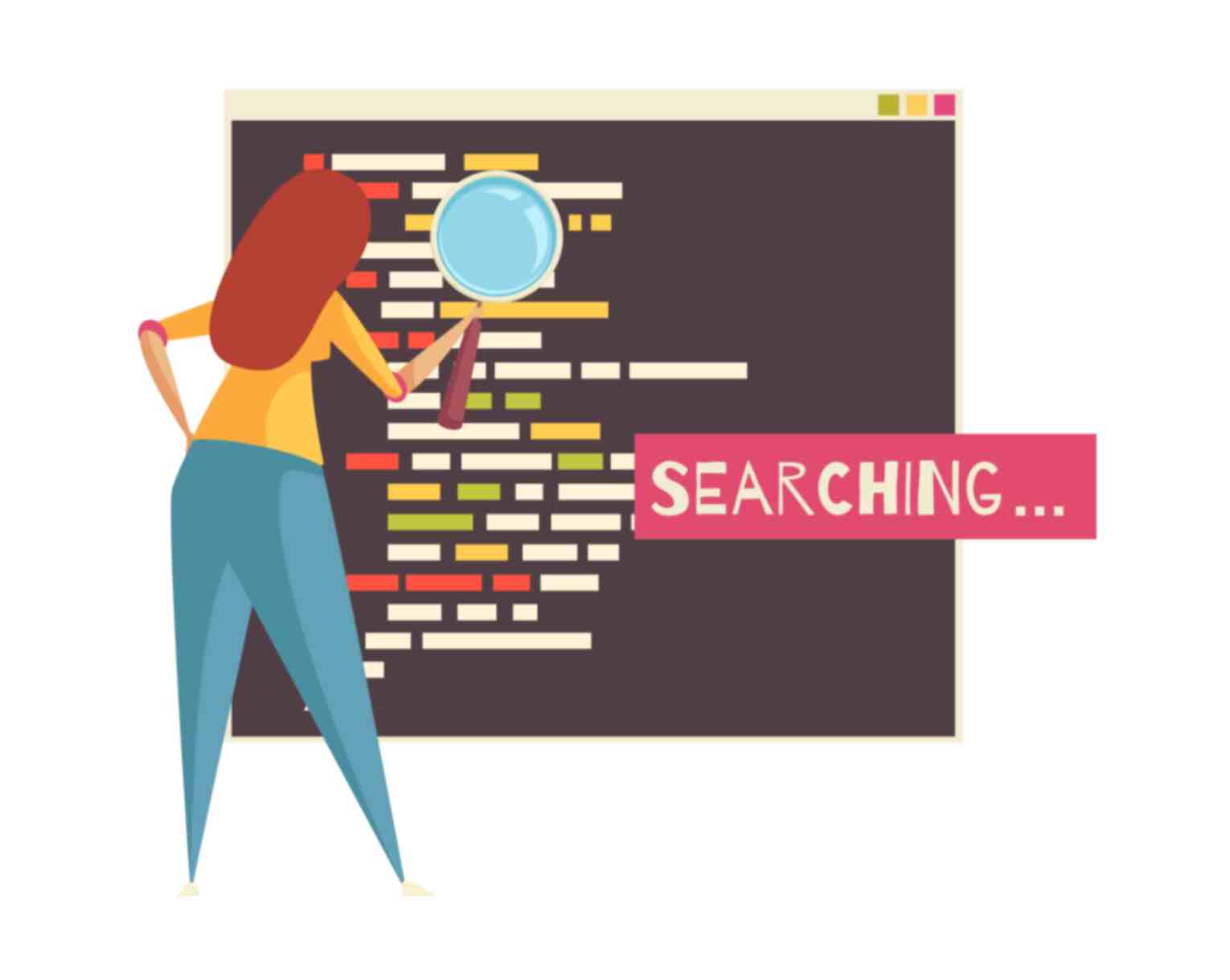
Introduction
Binary Search is a powerful and efficient algorithm used for searching within a sorted dataset. Whether you’re a novice programmer or a seasoned developer, understanding Binary Search is essential. In this comprehensive guide, we’ll demystify Binary Search and walk you through its Python implementation.
“Binary Search: Your trusty magnet for finding needles in haystacks.” — Anonymous Programmer
What is Binary Search?
Binary Search is a divide-and-conquer algorithm that expertly locates a target value within a sorted dataset. It thrives by iteratively splitting the search interval in half and eliminating the portion that doesn’t harbor the target value.
Visual Representation
Let’s visualize how Binary Search works with a sorted list of numbers:
Index | 0 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 |
---|---|---|---|---|---|---|---|---|---|---|
Value | 2 | 4 | 7 | 11 | 19 | 25 | 30 | 50 | 60 | 75 |
Imagine we want to find the value 30
. We’ll start with the entire list and repeatedly divide it in half until we find the target.
The Binary Search Process
- Initiate with the complete sorted dataset.
- Identify the middle element (in this instance,
7
). - Compare the middle element with the target (
30
). - As
30
surpasses7
, eliminate the left dataset half. - What remains is the right half:
[11, 19, 25, 30]
. - Repeat the procedure with the remaining data.
- Spot the middle element (
25
) and match it with the target (30
). -
30
surpasses25
, necessitating the removal of the left half once more. - Ultimately, we’re left with
[30]
, having successfully located our target!
Binary Search is incredibly efficient, making it ideal for large datasets.
Binary Search Flowchart
[Start]
Input: sorted_list, target_value
Find the middle element: mid = (left + right) // 2
Compare the middle element with the target value:
If arr[mid] == target_value:
Return mid
Else if arr[mid] < target_value:
Search the right half of the sorted list: left = mid + 1
Else:
Search the left half of the sorted list: right = mid - 1
[End]
Let’s Code!
(Python Program)
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 # Target not found
# Example usage:
sorted_list = [2, 4, 7, 11, 19, 25, 30, 50, 60, 75]
target_value = 30
result = binary_search(sorted_list, target_value)
print(f'Target {target_value} found at index {result}' if result != -1 else 'Target not found')
Javascript Program
function binarySearch(arr, target) {
let left = 0;
let right = arr.length - 1;
while (left <= right) {
let mid = Math.floor((left + right) / 2);
if (arr[mid] === target) {
return mid;
} else if (arr[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1; // Target not found
}
// Example usage:
const sortedList = [2, 4, 7, 11, 19, 25, 30, 50, 60, 75];
const targetValue = 30;
const result = binarySearch(sortedList, targetValue);
console.log(
result !== -1
? `Target ${targetValue} found at index ${result}`
: "Target not found"
);
Section 4: Pros and Cons
Pros
- Speed: Binary Search excels with large datasets.
- Simplicity: The algorithm is straightforward to comprehend and implement.
- Versatility: Binary Search can be applied to various sorted data structures.
Cons
- Sorted Data: Binary Search requires pre-sorted data.
- Limited to Static Data: Unsuitable for dynamic datasets.
Section 5: Real-World Examples
Binary Search finds applications in numerous scenarios:
-
Phonebook Lookup: Navigating a vast phonebook sorted by last names is made swift and efficient with Binary Search.
-
Library Catalog: In a well-organized library, Binary Search assists in locating books by author, title, or genre.
-
Dictionary Search: Quickly find word definitions and meanings in thick dictionaries.
-
E-commerce Sorting: When shopping online, Binary Search filters products by price range, brand, or customer reviews.
-
Game Development: Games employ Binary Search for inventory item retrieval, enemy identification, and player position tracking.
Conclusion
Binary Search, an algorithm that’s powerful and intuitive, is essential for efficient searching. By following these sections and exploring real-world examples, you’ll master Binary Search. Stay tuned for future articles offering advanced techniques and tips.
Explore More Topics
Happy coding and searching!