- Published on
Mastering Linear Search: A Comprehensive Guide for Efficient Searching
- Authors
-
-
- Name
- Jitendra M
- @_JitendraM
-
Table of contents:
-
Introduction
-
What is Linear Search?
-
Example
-
The Linear Search Process
-
Linear Search Flowchart
-
Let’s Code! (Python Program)
- Pros and Cons
-
Real-World Examples
-
Conclusion
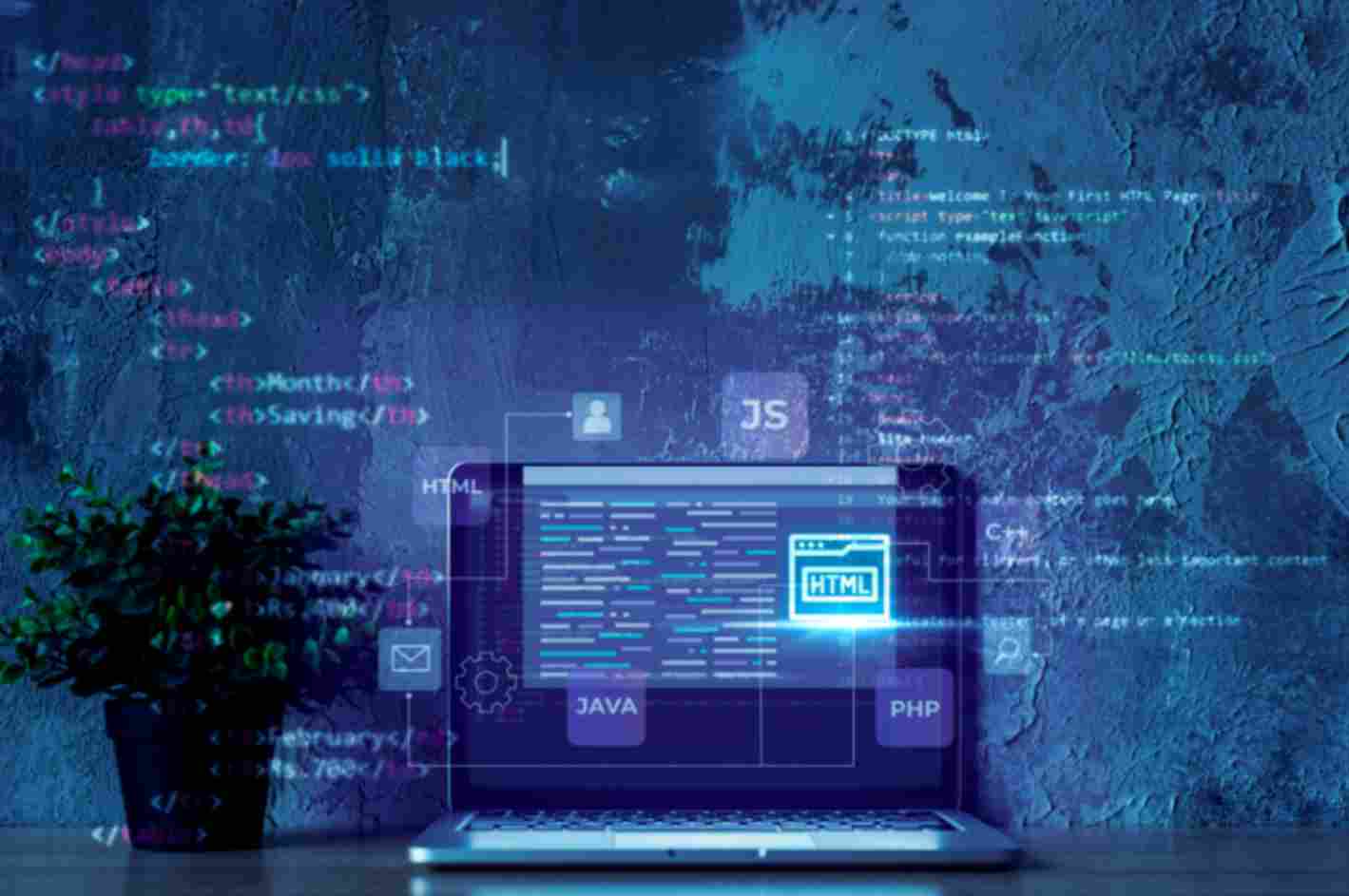
Introduction
In the world of algorithms, one of the fundamental techniques every programmer encounters is Linear Search, also known as Sequential Search. Whether you’re just starting your coding journey or you’re a seasoned developer, understanding Linear Search is paramount. In this detailed guide, we’ll demystify Linear Search and guide you through its Python implementation.
“Linear Search: Your trusty compass for navigating data landscapes.” — Anonymous Programmer
What is Linear Search?
At its core, Linear Search is a straightforward and intuitive searching algorithm. It scans through a dataset element by element, in a sequential manner, looking for a specific target value. It’s the most basic and rudimentary form of searching and serves as the foundation for more complex search algorithms.
Example
Let’s begin by visualizing how Linear Search operates with a simple example. Imagine we have an unsorted list of numbers:
[5, 12, 8, 3, 22, 15, 10, 6, 18, 9]
Our goal is to find a specific value, say 15
, within this list. The Linear Search algorithm will start from the beginning and traverse through the list one element at a time until it finds the target.
The Linear Search Process
Here’s a step-by-step breakdown of how Linear Search works:
-
Start at the beginning: We initiate our search by starting at the first element of the dataset.
-
Compare elements: We compare the current element with our target value (in this case,
15
). -
Found it!: If the current element matches our target, we’ve successfully found what we were looking for.
-
Not a match: If the current element doesn’t match the target, we move on to the next element and repeat the comparison.
-
Repeat until found: We continue this process until we either find the target or reach the end of the dataset.
Linear Search is a relatively simple and intuitive method, making it suitable for small datasets where efficiency is not a critical concern.
Linear Search Flowchart
Start
Input: Array of elements (arr[]), Search Element (target)
Step 1: Initialize a variable 'found' to false (found = false)
Step 2: Initialize a variable 'index' to -1 (index = -1)
Step 3: Start Loop
a. Check if the current index is equal to the length of the array (index == length of arr[])
b. If true, go to Step 4 (Element not found)
c. If false, go to Step 5
Step 4: Element not found
a. Display "Element not found in the array"
b. End
Step 5: Check the current element in the array
a. If the current element is equal to the target element (arr[index] == target), go to Step 6
b. If false, go to Step 7
Step 6: Element found
a. Set 'found' to true (found = true)
b. Set 'index' to the current index (index = current index)
c. Display "Element found at index: index"
d. End
Step 7: Move to the next element in the array
a. Increment the 'index' by 1 (index = index + 1)
b. Go back to Step 3 (Repeat the loop)
End
Let’s Code! (Python Program)
Now that we’ve understood the theory behind Linear Search, let’s dive into its Python implementation. Below is a Python program that demonstrates how Linear Search can be coded:
def linear_search(arr, target):
for index, element in enumerate(arr):
if element == target:
return index
return -1 # Target not found
# Example usage:
dataset = [5, 12, 8, 3, 22, 15, 10, 6, 18, 9]
target_value = 15
result = linear_search(dataset, target_value)
print(f'Target {target_value} found at index {result}' if result != -1 else 'Target not found')
Pros and Cons
Pros
- Simplicity: Linear Search is easy to understand and implement, making it suitable for beginners.
- Versatility: It can be used on both sorted and unsorted datasets, making it adaptable to various scenarios.
- Useful for Small Datasets: Linear Search is efficient for smaller lists where the overhead of more complex algorithms is unnecessary.
Cons
- Inefficiency with Large Datasets: For extensive data sets, Linear Search becomes slow and inefficient as it checks each element sequentially.
- Sequential Scanning: Linear Search scans elements one by one, which makes it less suitable for large-scale databases where faster search algorithms are preferred.
Real-World Examples
Linear Search finds practical applications in various real-world scenarios. Here are a few instances where Linear Search proves its usefulness:
-
Phonebook Lookup: Think about a vast phonebook sorted by names. When you want to find someone’s contact information, Linear Search allows you to quickly navigate through the pages, one by one, until you discover the desired entry. It’s a classic example of using Linear Search for searching unsorted data.
-
Library Catalog: In a well-organized library, Linear Search helps librarians and patrons locate books based on author names, book titles, or genres. Even with large book collections, Linear Search efficiently guides you to the right shelf.
-
Dictionary Search: When you’re flipping through a thick dictionary to find the definition of a word, you’re essentially performing a Linear Search. You start from the beginning and proceed page by page until you locate the word you’re looking for.
-
E-commerce Sorting: While shopping online, you often want to filter products based on specific criteria such as price range, brand, or customer reviews. Linear Search helps you narrow down your options by scanning through the product list sequentially.
-
Game Development: Linear Search is also present in the realm of game development. Games utilize Linear Search for tasks like identifying inventory items, searching for specific characters or objects, and tracking the player’s position in certain game scenarios.
These real-world examples showcase how Linear Search plays a pivotal role in simplifying everyday tasks and optimizing search operations in various domains.
Conclusion
In conclusion, Linear Search may be a simple and straightforward algorithm, but its importance in the world of computer science and problem-solving cannot be understated. It serves as the foundation for more complex search algorithms and is a valuable tool, especially in scenarios involving small datasets or unsorted data.
As you’ve explored this comprehensive guide, you’ve gained a deeper understanding of Linear Search, its principles, and its real-world applications. Remember that while Linear Search is efficient for specific use cases, it may not be the best choice for large datasets where more advanced search algorithms shine.
Stay tuned for more enlightening articles, tutorials, and insights on the ever-evolving landscape of technology and programming. Continue to expand your knowledge, and happy coding!