- Published on
Binary Search Trees: Structure, Operations, and Applications
- Authors
-
-
- Name
- Jitendra M
- @_JitendraM
-
Table of contents:
-
Introduction
-
What is a Binary Search Tree (BST)?
- How BSTs Work
-
Properties of BSTs
-
Time Complexity
-
Pseudo Code for Binary Search Trees
-
Example
-
Binary Search Tree in Python
-
Binary Search Tree in Java
- Pros and Cons
-
Advanced BST Topics
-
Choosing the Right Data Structure
-
Comparison with Other Tree Algorithms
-
Real-World Applications
-
Conclusion
-
Exlpore more topics
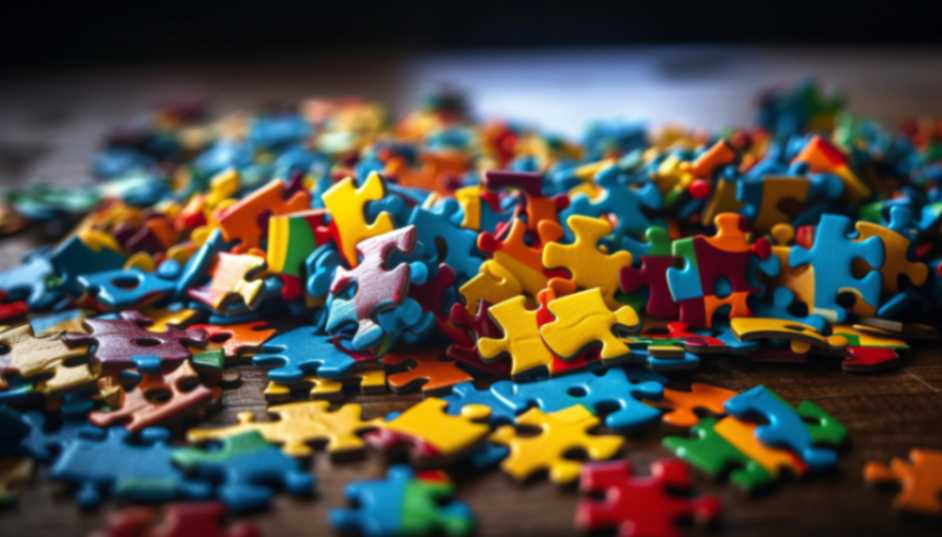
Introduction
Binary Search Trees (BSTs) are versatile and efficient data structures used in computer science and various applications. They offer fast and organized data retrieval and are essential for tasks like searching, sorting, and maintaining hierarchical data. In this article, we’ll explore what BSTs are, how they work, their properties, time complexity, pros and cons, and real-world applications.
What is a Binary Search Tree (BST)?
A Binary Search Tree (BST) is a hierarchical data structure that organizes data in a tree-like form. Each node in a BST contains a value or key and has at most two child nodes: a left child and a right child. The key property of a BST is that for every node:
- All nodes in its left subtree have values less than the node’s value.
- All nodes in its right subtree have values greater than the node’s value.
This property makes BSTs particularly useful for efficient searching and sorting operations.
How BSTs Work
BSTs rely on their hierarchical structure and the key property to efficiently store and retrieve data. Here’s how some common operations work:
Insertion
When inserting a new value into a BST:
- Compare the value with the root node.
- If it’s less than the root, move to the left subtree; if greater, move to the right.
- Repeat this process until you find an empty spot (a leaf node) to insert the new value.
Search
Searching in a BST is similar to insertion:
- Compare the target value with the root node.
- If it matches, you’ve found the value.
- If it’s less than the root, search in the left subtree; if greater, search in the right subtree.
- Repeat until you find the value or reach a leaf node (indicating the value is not in the tree).
Deletion
Deleting a node in a BST requires careful handling to maintain the BST property:
- If the node to delete is a leaf or has only one child, simply remove it.
- If it has two children, find the in-order predecessor or successor (the node with the closest value), replace the node to delete with it, and then delete the predecessor or successor.
Properties of BSTs
BSTs have several properties that make them valuable data structures:
-
Fast Search: Thanks to their hierarchical structure, BSTs offer fast searching with an average time complexity of
O(log n)
for balanced trees. -
Ordered Data: BSTs naturally store data in sorted order, making it easy to retrieve data in ascending or descending order.
-
Efficient Insertion and Deletion: For balanced BSTs, insertion and deletion also have an average time complexity of
O(log n)
.
Time Complexity
BSTs offer efficient time complexities for search, insertion, and deletion operations. However, these complexities depend on the tree’s balance. In the worst case (unbalanced tree), the time complexity can be O(n)
, where n is the number of nodes. To maintain balanced trees, various self-balancing BSTs, like AVL trees and Red-Black trees, are used.
Pseudo Code for Binary Search Trees
Here’s a simple pseudo code representation of a BST insertion operation:
function insert(root, value)
if root is null
return createNewNode(value)
if value < root.value
root.left = insert(root.left, value)
else if value > root.value
root.right = insert(root.right, value)
return root`
Example
Let’s consider a simple example to better understand how a BST works:
10
/ \
5 15
/ \ \
3 8 20`
- We start with an empty tree and insert the values 10, 5, 15, 3, 8, and 20 in that order.
- The first insertion (10) creates the root node.
- Subsequent insertions follow the rules: smaller values to the left, larger values to the right.
- After all insertions, the tree maintains the BST property.
Binary Search Tree in Python
Here’s a simple Python implementation of a Binary Search Tree:
class TreeNode:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
def insert(root, value):
if root is None:
return TreeNode(value)
if value < root.value:
root.left = insert(root.left, value)
else:
root.right = insert(root.right, value)
return root
Binary Search Tree in Java
And here’s the equivalent Java implementation:
class Node {
int key;
Node left, right;
public Node(int item) {
key = item;
left = right = null;
}
}
public class BinarySearchTree {
Node root;
BinarySearchTree() {
root = null;
}
void insert(int key) {
root = insertRec(root, key);
}
Node insertRec(Node root, int key) {
if (root == null) {
root = new Node(key);
return root;
}
if (key < root.key)
root.left = insertRec(root.left, key);
else if (key > root.key)
root.right = insertRec(root.right, key);
return root;
}
boolean search(int key) {
return searchRec(root, key);
}
boolean searchRec(Node root, int key) {
if (root == null)
return false;
if (root.key == key)
return true;
if (key < root.key)
return searchRec(root.left, key);
return searchRec(root.right, key);
}
public static void main(String[] args) {
BinarySearchTree tree = new BinarySearchTree();
tree.insert(50);
tree.insert(30);
tree.insert(20);
tree.insert(40);
tree.insert(70);
tree.insert(60);
tree.insert(80);
if (tree.search(40))
System.out.println("Key 40 found in the BST");
else
System.out.println("Key 40 not found in the BST");
}
}
Pros and Cons
Pros:
-
Efficiency: BSTs provide fast search, insertion, and deletion operations when balanced.
-
Ordered Data: Data is naturally sorted in a BST, making it suitable for tasks that require sorted data.
-
Versatility: BSTs can be used for a wide range of applications, from database indexing to spell checking.
Cons:
- Balancing: Maintaining balance in a BST can be challenging, and unbalanced trees can lead to performance degradation.
Advanced BST Topics
Advanced BST topics include self-balancing BSTs like AVL trees. These structures automatically adjust their shape during insertions and deletions to maintain balance, ensuring efficient operations.
Choosing the Right Data Structure
When choosing a data structure, consider your specific application needs. Compare BSTs with other data structures like hash tables and linked lists to determine the best fit.
Comparison with Other Tree Algorithms
BSTs are just one type of tree structure. Other tree algorithms, such as B-trees and Red-Black trees, have their own advantages and use cases. Comparing these algorithms can help you select the most appropriate one for your application.
Real-World Applications
BSTs have applications in various fields:
-
Database Indexing: BSTs are used in database management systems to efficiently search and retrieve data.
-
Spell Checking: BSTs help in quickly checking if a word is spelled correctly by storing a dictionary of words.
-
File Systems: Some file systems use BSTs to manage and locate files efficiently.
-
Optimization Algorithms: In optimization problems, BSTs can be used to find the best solutions efficiently.
Conclusion
Binary Search Trees are fundamental data structures with a wide range of applications. They provide efficient searching, insertion, and deletion operations, making them valuable in computer science and real-world scenarios. Understanding BSTs and their properties can help you make informed decisions when designing algorithms and systems.
Now that you’ve learned about Binary Search Trees, consider exploring more advanced topics like self-balancing BSTs or other tree-based data structures.