- Published on
Decision Trees: A Powerful and Versatile Machine Learning Algorithm
- Authors
-
-
- Name
- Jitendra M
- @_JitendraM
-
Table of contents:
-
Introduction
-
How Decision Trees Work
-
Python Implementation of Decision Trees
-
Example: Using Decision Trees for Iris Classification
- Pros and Cons
-
Hyperparameters and Tuning
-
Types of Decision Trees
-
Limitations
-
Real-World Use Cases
- Comparison with Other Machine Learning Algorithms
-
Explore More Topics
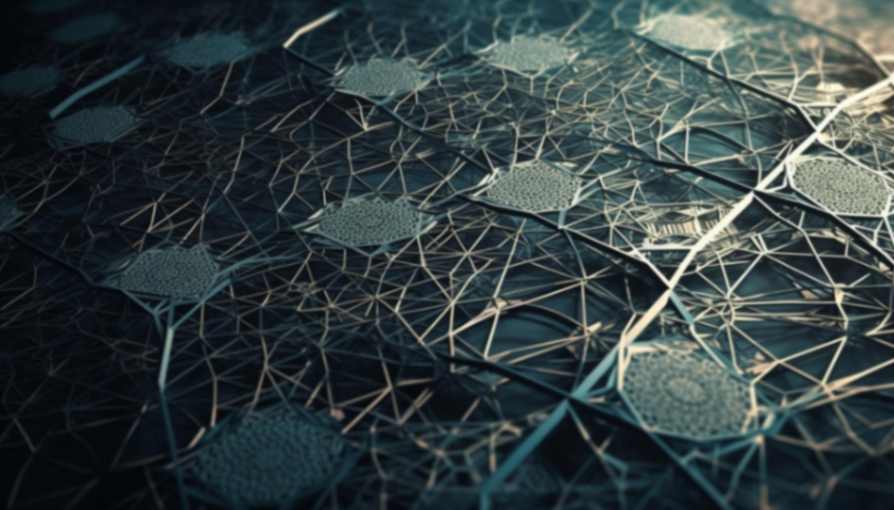
Decision Trees are a powerful and versatile machine learning algorithm used for both classification and regression tasks. They are widely employed in various fields, including finance, healthcare, and marketing. In this article, we will delve into how Decision Trees work, discuss their pros and cons, offer real-world use cases, explore hyperparameters and different types of Decision Trees, and address their limitations.
Introduction
A Decision Tree is a hierarchical structure that resembles a tree. It consists of nodes that represent decisions or tests on specific features, branches that lead to subsequent decisions, and leaves that represent the outcomes or predictions. Decision Trees are known for their interpretability and ability to handle both categorical and numerical data.
How Decision Trees Work
The construction of a Decision Tree involves selecting the best attribute to split the data at each node. This selection is based on criteria such as Gini impurity or information gain. Here’s a step-by-step overview of how Decision Trees work:
- Start with the entire dataset at the root node.
- Choose the best attribute to split the data based on a criterion (e.g., Gini impurity or information gain).
- Create child nodes for each possible outcome of the selected attribute.
- Repeat steps 1-3 for each child node until one of the stopping criteria is met (e.g., maximum depth, minimum samples per leaf).
- Assign the most common class label (classification) or the average value (regression) of the samples in a leaf node.
Python Implementation of Decision Trees
Let’s implement a Decision Tree classifier in Python using the popular scikit-learn library:
from sklearn.tree import DecisionTreeClassifier
# Create a Decision Tree classifier
clf = DecisionTreeClassifier()
# Train the classifier on a dataset
clf.fit(X_train, y_train)
# Make predictions
predictions = clf.predict(X_test)
In this code snippet, we create a Decision Tree classifier, train it on a dataset (X_train
, y_train
), and then use it to make predictions.
Example: Using Decision Trees for Iris Classification
Let’s demonstrate the use of Decision Trees with a classic example: classifying iris flowers based on their sepal length and width. We will use the Iris dataset provided by scikit-learn.
from sklearn.datasets import load_iris
from sklearn.tree import DecisionTreeClassifier
from sklearn.model_selection import train_test_split
# Load the Iris dataset
iris = load_iris()
X = iris.data
y = iris.target
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# Create a Decision Tree classifier
clf = DecisionTreeClassifier()
# Train the classifier
clf.fit(X_train, y_train)
# Make predictions
predictions = clf.predict(X_test)
In this example, we use Decision Trees to classify iris flowers into different species based on their sepal characteristics.
Pros and Cons
Pros:
- Interpretability: Decision Trees are easy to visualize and interpret, making them useful for explaining model predictions.
- Handling Nonlinear Relationships: They can capture nonlinear relationships in data.
- Handling Mixed Data Types: Decision Trees can handle both categorical and numerical data.
Cons:
- Overfitting: Decision Trees are prone to overfitting, especially when the tree is deep.
- Instability: Small variations in data can result in different tree structures.
- Bias: Decision Trees can be biased if some classes dominate.
Hyperparameters and Tuning
To avoid overfitting, Decision Trees have hyperparameters that can be tuned, such as maximum depth and minimum samples per leaf. Tuning these hyperparameters can help optimize the model’s performance.
Types of Decision Trees
There are different types of Decision Trees, including classification trees (for categorical outcomes) and regression trees (for numerical outcomes). Understanding the type of problem you’re solving helps in choosing the right type of Decision Tree.
Limitations
Despite their versatility, Decision Trees have limitations:
-
Overfitting: Decision Trees can easily overfit noisy data, leading to poor generalization on unseen data. Proper pruning and hyperparameter tuning are necessary to mitigate this issue.
-
Instability: Small variations in the data can result in different tree structures. This instability can be addressed by using ensemble methods like Random Forests.
Real-World Use Cases
Decision Trees find applications in various real-world scenarios:
-
Credit Scoring: Banks use Decision Trees to assess the creditworthiness of loan applicants.
-
Medical Diagnosis: Healthcare professionals employ Decision Trees to assist in diagnosing diseases based on patient data.
-
Recommendation Systems: Companies like Amazon use Decision Trees to recommend products to users based on their preferences.
Comparison with Other Machine Learning Algorithms
Decision Trees vs. Random Forests
- Random Forests are an ensemble method that uses multiple Decision Trees to improve accuracy and reduce overfitting.
Decision Trees vs. Support Vector Machines (SVM)
- Support Vector Machines are effective for high-dimensional data and binary classification but may lack interpretability compared to Decision Trees.
In summary, Decision Trees are versatile and interpretable machine learning models suitable for various tasks. Careful tuning of hyperparameters and understanding the problem type can enhance their performance.