- Published on
Red-Black Trees: Balanced Data Structures
- Authors
-
-
- Name
- Jitendra M
- @_JitendraM
-
Table of contents:
-
Introduction
-
What Are Red-Black Trees?
-
Red-Black Tree Operations
-
Time Complexity
-
Storage Complexity
-
Pseudo Code for Red-Black Trees
-
Example
-
Red-Black Tree in Python
-
Red-Black Tree in C++
-
Comparison with Other Related Algorithms
- Pros and Cons
-
Real-World Applications
-
Conclusion
-
Explore more
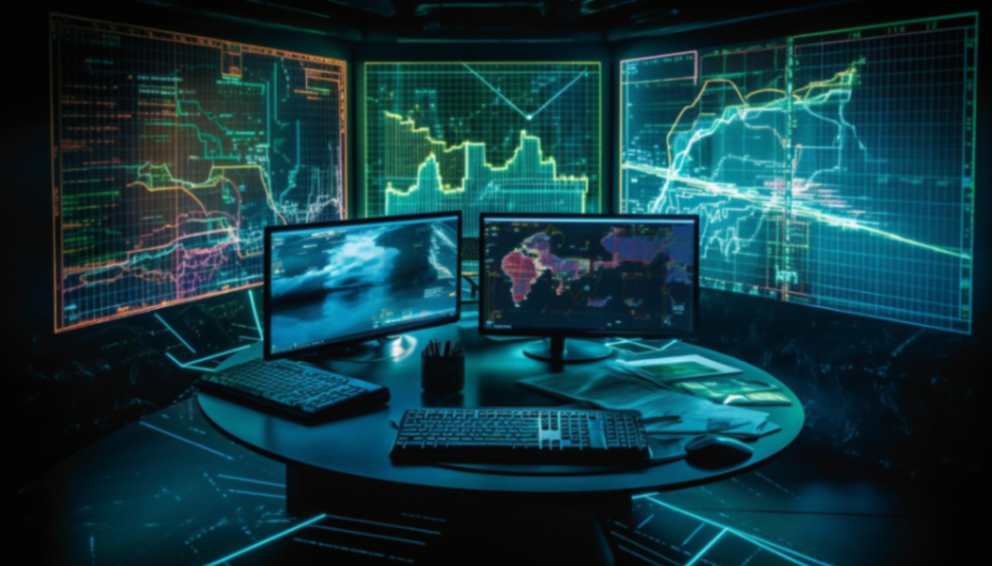
Introduction
Red-Black Trees are a type of self-balancing binary search tree (BST) that offers an efficient and balanced solution for storing and managing data. They ensure that the tree remains relatively balanced, preventing degeneration into a skewed structure. In this article, we’ll delve into Red-Black Trees, exploring their properties, operations, time complexity, storage complexity, C++ and Python implementations, and real-world applications. We’ll also compare Red-Black Trees with related algorithms to understand their advantages.
What Are Red-Black Trees?
Red-Black Trees are binary search trees with the following properties:
- Node Colors: Each node is colored either red or black.
- Root Property: The root is always black.
- Red Property: Red nodes cannot have red child nodes but can have black child nodes.
- Black Depth Property: For each node, any path from that node to its descendant leaves must have the same number of black nodes. This ensures the tree remains relatively balanced.
Red-Black Tree Operations
Red-Black Trees support standard binary search tree operations such as insertion, deletion, and search. However, these operations come with additional rules to maintain the tree’s balance.
Insertion:
- Perform a standard BST insertion.
- Color the new node red.
- Ensure that no two consecutive red nodes exist along any path.
- Recolor or rotate the tree to satisfy the Red-Black properties.
Deletion:
- Perform a standard BST deletion.
- If a red node is deleted, no rules are violated.
- If a black node is deleted, the tree may violate the Black Depth Property, so additional operations like recoloring or rotation are needed to restore balance.
Searching:
- Red-Black Trees support standard binary search for data retrieval, similar to other binary search trees.
Time Complexity
Red-Black Trees offer efficient time complexity for various operations:
-
Search:
O(log n)
-
Insertion:
O(log n)
-
Deletion:
O(log n)
These time complexities hold as long as the tree remains balanced. The logarithmic behavior ensures fast operations for large datasets.
Storage Complexity
Red-Black Trees are memory-efficient, with a storage complexity of O(n)
, where n
is the number of nodes in the tree.
Pseudo Code for Red-Black Trees
Here’s a simple pseudo code representation of a Red-Black Tree insertion operation:
function insert(root, value)
if root is null
return createNewNode(value)
if value < root.value
root.left = insert(root.left, value)
else if value > root.value
root.right = insert(root.right, value)
return root
Example
To illustrate Red-Black Trees, consider the following tree:
10 (Black)
/ \
5 (Red) 15 (Red)
/ \ / \
3 (Black) 12 (Black) 17 (Black)
This Red-Black Tree adheres to the tree properties. The black depth from the root to any leaf node remains consistent, and no consecutive red nodes exist along any path.
Red-Black Tree in Python
Here’s an example of a Python Red-Black Tree implementation for insertion and searching:
# Sample Python Red-Black Tree Implementation
class Node:
def __init__(self, data):
self.data = data
self.color = "RED"
self.left = None
self.right = None
self.parent = None
class RedBlackTree:
def __init__(self):
self.NIL_LEAF = Node(0)
self.NIL_LEAF.color = "BLACK"
self.NIL_LEAF.left = None
self.NIL_LEAF.right = None
self.root = self.NIL_LEAF
Red-Black Tree in C++
Here’s an example of a C++ Red-Black Tree implementation for insertion and searching:
// Sample C++ Red-Black Tree Implementation
#include <bits/stdc++.h>
using namespace std;
enum Color { RED, BLACK };
struct Node {
int data;
Color color;
Node *left, *right, *parent;
Node(int data) : data(data) {
parent = left = right = nullptr;
color = RED;
}
};
class RedBlackTree {
Node *root;
public:
// Functions for insertion, searching, and other operations
};
int main() {
RedBlackTree tree;
// Perform Red-Black Tree operations
return 0;
}
Comparison with Other Related Algorithms
Red-Black Trees are related to other self-balancing binary search trees like AVL trees. Comparing these algorithms can help you choose the most appropriate one for your application.
Pros and Cons
Pros
- Efficiency: Red-Black Trees provide fast search, insertion, and deletion operations when balanced.
- Balanced Structure: Red-Black Trees automatically maintain their balance, ensuring efficient operations.
Cons
- Implementation Complexity: Implementing Red-Black Trees requires careful consideration of the balancing rules.
- Storage Overhead: Storing color information for each node adds some overhead.
Real-World Applications
Red-Black Trees are applied in various scenarios:
-
C++ STL Sets and Maps: In the C++ Standard Template Library, sets and maps often use Red-Black Trees due to their balance and efficiency.
-
Java TreeMap: Java’s TreeMap implementation relies on Red-Black Trees, providing sorted map functionality.
-
Linux Virtual File System (VFS): Red-Black Trees are employed in the Linux kernel’s VFS subsystem for file directory management.
-
Database Indexing: Red-Black Trees are used in database systems for indexing, ensuring efficient data retrieval.
Conclusion
Red-Black Trees are a fundamental data structure that helps maintain balance in binary search trees. Their self-balancing properties ensure efficient operations even with dynamic data. Understanding Red-Black Trees is valuable for algorithm design, database management, and other applications.
Now that you’ve explored Red-Black Trees, consider their use in scenarios where balanced binary search trees are essential for optimal performance.