- Published on
Understanding Bubble Sort Algorithm: A Complete Guide
- Authors
-
-
- Name
- Jitendra M
- @_JitendraM
-
Table of contents:
-
Introduction
-
How Bubble Sort Works
-
Example: Sorting a List
-
Block diagram
-
Python Implementation of Bubble Sort
- Advantages and Disadvantages
-
Real-World Use Cases
- Comparison with Other Sorting Algorithms
-
Conclusion
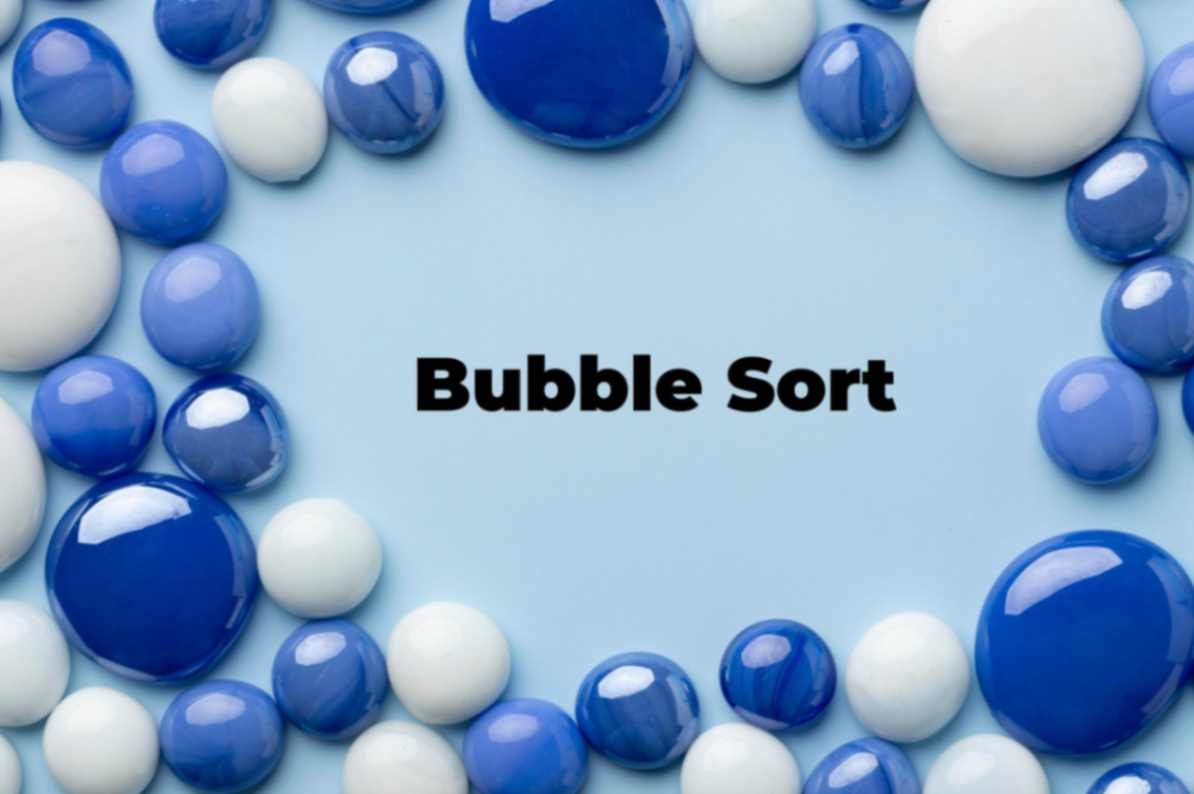
Introduction
Bubble Sort is a simple and widely used sorting algorithm in computer science. It belongs to the family of comparison-based sorting algorithms and is known for its simplicity and ease of understanding. In this guide, we’ll explore how Bubble Sort works, its advantages and disadvantages, provide real-world examples of where it can be used, and compare it with other sorting algorithms.
How Bubble Sort Works
Bubble Sort gets its name from the way smaller elements “bubble” to the top of the list during each pass. Here’s a step-by-step explanation of how Bubble Sort works:
- Start at the beginning of the list.
- Compare the first two elements. If the first element is larger than the second, swap them.
- Move to the next pair of elements and repeat step 2.
- Continue this process until you reach the end of the list. At this point, the largest element is guaranteed to be at the end.
- Repeat steps 1-4 for the remaining unsorted portion of the list, excluding the last element.
- Continue this process until the entire list is sorted.
Example: Sorting a List
Let’s walk through a simple example of using Bubble Sort to sort a list of numbers:
Suppose we have an unsorted list: [5, 2, 9, 3, 6]
.
Step 1: Start with the first element and compare it to the next element. If the first element is greater, swap them.
- Initial list:
[5, 2, 9, 3, 6]
- After the first pass:
[2, 5, 9, 3, 6]
Step 2: Continue comparing and swapping adjacent elements until the largest element “bubbles up” to the end of the list.
- After the second pass:
[2, 5, 3, 9, 6]
- After the third pass:
[2, 5, 3, 6, 9]
Step 3: Repeat the process for the remaining unsorted portion of the list.
- After the fourth pass:
[2, 3, 5, 6, 9]
The list is now sorted in ascending order.
Block diagram
[Start]
-> [Is the list sorted?]
--> Yes: [End]
--> No: [Compare the first two elements and swap them if necessary]
-> [Move to the next pair of elements]
-> [Repeat steps 2-3 until the end of the list is reached]
-> [Repeat steps 2-4 for the remaining unsorted portion of the list, excluding the last element]
-> [Repeat steps 2-5 until the entire list is sorted]
[End]
Python Implementation of Bubble Sort
Let’s implement Bubble Sort in Python:
def bubble_sort(arr):
n = len(arr)
for i in range(n):
swapped = False
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j] # Swap the elements
swapped = True
if not swapped:
# If no two elements were swapped in the inner loop, the array is already sorted
break
return arr
Advantages and Disadvantages
Advantages:
- Simplicity: Bubble Sort is easy to understand and implement.
- Space Complexity: It has low space complexity as it doesn’t require additional memory for sorting.
Disadvantages:
- Inefficiency: Bubble Sort has poor time complexity, especially for large datasets.
- Not Suitable for Large Lists: It is not efficient for sorting large lists or arrays.
Real-World Use Cases
Bubble Sort, while not suitable for large datasets, can be applied in various real-world scenarios, such as:
- Educational Purposes: It is often used to teach the concept of sorting algorithms due to its simplicity.
- Small Datasets: Bubble Sort can be adequate for sorting small lists or arrays.
- Sorting Partially Sorted Lists: If a list is already partially sorted, Bubble Sort can be faster than other algorithms.
Comparison with Other Sorting Algorithms
Bubble Sort is a simple sorting algorithm, but it has limitations compared to more efficient algorithms like Quick Sort and Merge Sort.
Bubble Sort vs. Quick Sort:
- Quick Sort is more efficient than Bubble Sort in terms of time complexity, especially for large datasets.
- Quick Sort uses a divide-and-conquer approach, making it faster in practice.
- Bubble Sort has a worst-case time complexity of O(n^2), while Quick Sort has an average-case time complexity of O(n log n).
Bubble Sort vs. Merge Sort:
- Merge Sort is a stable, efficient sorting algorithm that outperforms Bubble Sort.
- Merge Sort divides the list into smaller sublists, sorts them, and then merges them, resulting in a time complexity of O(n log n).
- Bubble Sort’s time complexity remains O(n^2) in all cases.
In practice, Bubble Sort is rarely used for large datasets or performance-critical applications due to its inefficiency. More advanced sorting algorithms like Quick Sort and Merge Sort are preferred.
Conclusion
Bubble Sort is a straightforward sorting algorithm suitable for educational purposes and small datasets. However, it is not efficient for large-scale data sorting. Understanding how Bubble Sort works can provide valuable insights into sorting algorithms and computer science fundamentals.