- Published on
Mastering Job Scheduling Algorithms for Efficient Task Management
- Authors
-
-
- Name
- Jitendra M
- @_JitendraM
-
Table of contents:
-
Introduction
-
What is Job Scheduling?
-
How Does Job Scheduling Work?
-
Time Complexity
-
Space Complexity
-
Pseudo Code
-
Example
-
Python Implementation
-
C++ Implementation
-
Comparison with Other Algorithms
-
Pros and Cons
-
Real-World Applications
-
Challenges and Considerations
-
Conclusion
-
Explore More Topics
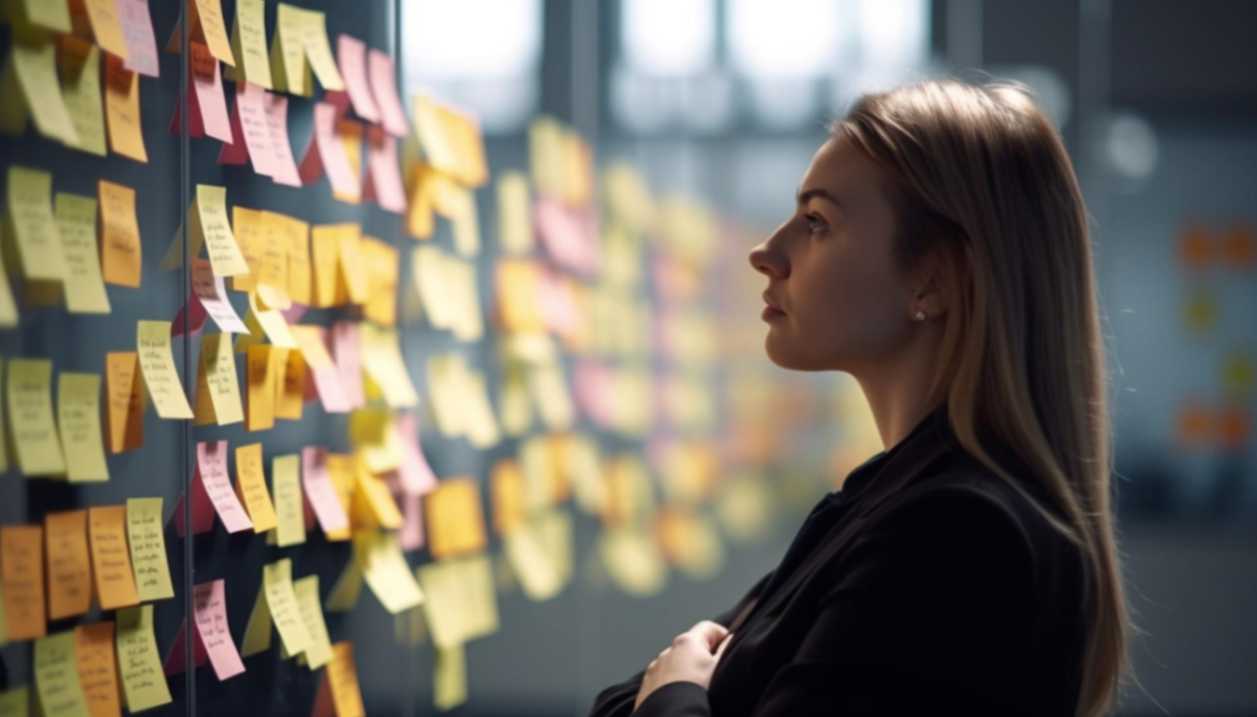
Introduction
Job scheduling plays a crucial role in optimizing resource utilization and managing tasks efficiently. In this article, we’ll delve into the intricacies of job scheduling algorithms, exploring their significance in computing systems and real-world applications.
What is Job Scheduling?
Job scheduling involves orchestrating the execution of tasks or jobs in a computing system. These jobs may have different priorities, execution times, and resource requirements. The primary goal is to enhance system performance by minimizing turnaround time, maximizing throughput, and ensuring fair allocation of resources.
How Does Job Scheduling Work?
Job scheduling algorithms determine the order in which jobs are executed based on specific criteria. These criteria may include job priority, execution time, deadlines, or a combination of factors. The choice of algorithm significantly influences system responsiveness and efficiency.
Time Complexity
The time complexity of job scheduling algorithms varies depending on the specific algorithm employed. Common algorithms, such as First-Come, First-Served (FCFS), Shortest Job Next (SJN), and Round Robin, have different time complexities. Understanding these complexities is vital for selecting the most suitable algorithm for a given scenario.
Space Complexity
Job scheduling algorithms generally have minimal space complexity, as they primarily involve managing queues or lists of jobs. The space required for these algorithms is typically proportional to the number of jobs in the system.
Pseudo Code
Let’s explore a basic pseudo code snippet for a Round Robin job scheduling algorithm:
function roundRobinScheduler(jobs, timeQuantum):
initialize empty queue
initialize time counter
while jobs are not empty:
currentJob = dequeue(jobs)
execute currentJob for timeQuantum
update time counter
if currentJob is not completed:
enqueue(currentJob, jobs) // reinsert the job
return
Example
Consider a set of jobs with varying execution times:
Job | Execution Time |
---|---|
A | 8 |
B | 4 |
C | 2 |
D | 5 |
Let’s apply the Round Robin algorithm with a time quantum of 3 units:
- A executes for 3 units (remaining time: 5).
- B executes for 3 units (remaining time: 1).
- C executes for 2 units (completed).
- D executes for 3 units (remaining time: 2).
- A executes for 3 units (remaining time: 2).
- D executes for 2 units (completed).
- A executes for 2 units (completed).
Python Implementation
def round_robin_scheduler(jobs, time_quantum):
queue = []
time_counter = 0
while jobs:
current_job = jobs.pop(0)
execution_time = min(time_quantum, current_job[1])
time_counter += execution_time
if current_job[1] > time_quantum:
current_job = (current_job[0], current_job[1] - time_quantum)
jobs.append(current_job)
print(f"Executing {current_job[0]} for {execution_time} units. Remaining time: {current_job[1]}")
return
C++ Implementation
#include <iostream>
#include <queue>
#include <vector>
void roundRobinScheduler(std::vector<std::pair<char, int>>& jobs, int timeQuantum) {
std::queue<std::pair<char, int>> jobQueue;
int timeCounter = 0;
while (!jobs.empty()) {
auto currentJob = jobs.front();
jobs.erase(jobs.begin());
int executionTime = std::min(timeQuantum, currentJob.second);
timeCounter += executionTime;
if (currentJob.second > timeQuantum) {
currentJob.second -= timeQuantum;
jobs.push_back(currentJob);
}
std::cout << "Executing " << currentJob.first << " for " << executionTime
<< " units. Remaining time: " << currentJob.second << std::endl;
}
}
int main() {
std::vector<std::pair<char, int>> jobs = {{'A', 8}, {'B', 4}, {'C', 2}, {'D', 5}};
int timeQuantum = 3;
roundRobinScheduler(jobs, timeQuantum);
return 0;
}
Comparison with Other Algorithms
Job scheduling algorithms vary in their approaches and suitability for different scenarios. While Round Robin ensures fairness and prevents starvation, algorithms like FCFS and SJN prioritize simplicity and may lead to suboptimal results in certain situations.
Pros and Cons
Pros:
- Fair allocation of CPU time in Round Robin.
- Simple implementation in FCFS.
- Low space complexity for most algorithms.
Cons:
- Round Robin may lead to higher turnaround time for longer jobs.
- FCFS lacks adaptability to varying job execution times.
- SJN may cause starvation for longer jobs.
Real-World Applications
Job scheduling algorithms find applications in a wide range of computing domains, including:
-
Operating Systems: Operating systems employ job scheduling algorithms to manage the execution of processes and tasks, ensuring efficient resource utilization and system responsiveness.
-
Cloud Computing: In cloud computing environments, job scheduling algorithms are crucial for allocating virtual resources to different user requests, optimizing resource utilization, and ensuring service quality.
-
Distributed Systems: Distributed systems rely on job scheduling algorithms to coordinate the execution of tasks across multiple nodes, ensuring efficient task completion and system-wide performance.
-
Network Protocols: Network protocols often incorporate job scheduling mechanisms to prioritize data packets and manage network congestion.
-
Embedded Systems: Embedded systems, such as those found in real-time applications, utilize job scheduling algorithms to meet strict timing constraints and ensure system stability.
Challenges and Considerations
Effective job scheduling in real-world scenarios poses several challenges:
-
Dynamic Workloads: Handling dynamic workloads, where the number and characteristics of jobs can change rapidly, requires adaptive scheduling algorithms.
-
Unpredictable Task Execution Times: Real-world tasks may have unpredictable execution times, leading to challenges in accurate scheduling and performance guarantees.
-
Fairness and Efficiency: Achieving a balance between fairness, ensuring equal access to resources, and efficiency, maximizing resource utilization, is a critical consideration in job scheduling.
-
Resource Heterogeneity: In distributed systems and cloud environments, resource heterogeneity, where resources have different capabilities and availability, adds complexity to scheduling decisions.
-
Fault Tolerance: Job scheduling algorithms need to be fault-tolerant, able to handle system failures and unexpected events without compromising performance or fairness.
These challenges necessitate the development of sophisticated scheduling algorithms and techniques that can adapt to dynamic environments, handle unpredictable task execution times, and ensure fairness and efficiency in complex systems.
Conclusion
Understanding job scheduling algorithms is essential for optimizing system performance and resource utilization. The choice of algorithm depends on the specific requirements and characteristics of the computing environment. As we explore more advanced topics and algorithms, the nuances of job scheduling become even more critical for achieving efficient and responsive systems.