- Published on
Understanding the k-Nearest Neighbors (k-NN) Algorithm
- Authors
-
-
- Name
- Jitendra M
- @_JitendraM
-
Table of contents:
-
Introduction
-
What is the k-NN Algorithm?
-
Python Implementation of k-NN
- Pros and Cons of k-NN
- Comparison with Other Algorithms
-
Example: Classifying Iris Flowers
-
Real-World Use Cases
-
Conclusion
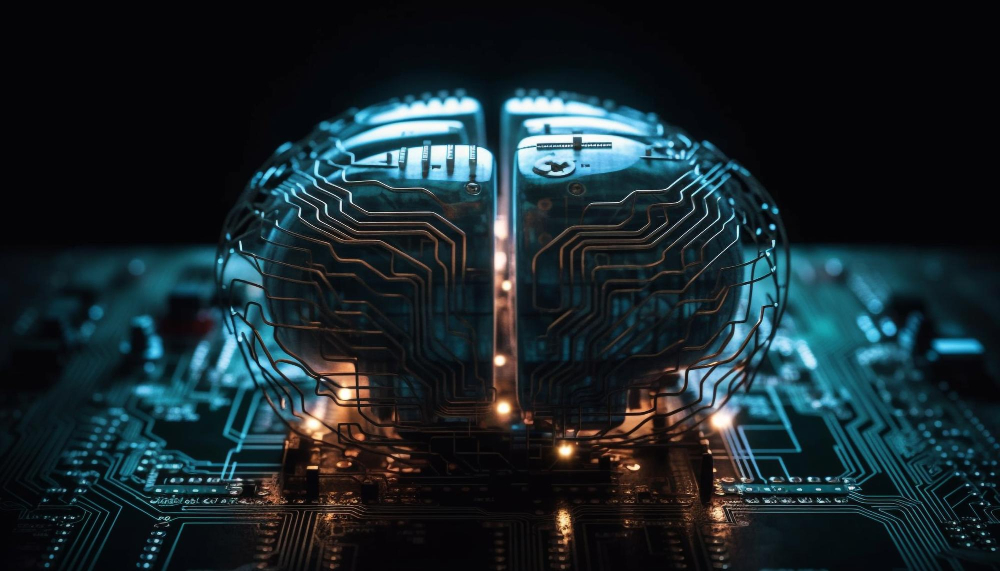
Introduction
In the realm of machine learning, the k-Nearest Neighbors (k-NN) algorithm is a versatile and intuitive approach used for classification and regression tasks. It belongs to the supervised learning category and is particularly helpful when you need to make predictions based on similarity measures between data points. In this guide, we’ll delve into the k-NN algorithm, explore its Python implementation, weigh its pros and cons, draw comparisons with other algorithms, provide a hands-on example with tabular visuals, and illustrate real-world use cases.
What is the k-NN Algorithm?
At its core, the k-NN algorithm relies on the principle that similar data points tend to belong to the same class or share similar numerical values. Understanding the k-NN algorithm is beneficial because it offers:
- Versatility: It can be used for various tasks, including classification, regression, and anomaly detection.
- Intuitiveness: The concept of proximity-based classification is easy to grasp.
- Applicability: It doesn’t require building an explicit model during training, making it ideal for dynamic datasets.
Here are the key components of the k-NN algorithm:
- k: A user-defined parameter that represents the number of nearest neighbors to consider.
- Distance Metric: A method for measuring the similarity or dissimilarity between data points. Common distance metrics include Euclidean distance, Manhattan distance, and more.
Python Implementation of k-NN
Let’s dive into Python and implement the k-NN algorithm using the scikit-learn library. Scikit-learn simplifies machine learning tasks and provides a convenient interface for k-NN.
# Importing necessary libraries
from sklearn.neighbors import KNeighborsClassifier
# Creating a k-NN classifier with k=3 and using Euclidean distance
knn = KNeighborsClassifier(n_neighbors=3)
# Fitting the model with training data
knn.fit(X_train, y_train)
# Making predictions on new data
predictions = knn.predict(X_test)
Pros and Cons of k-NN
Pros:
- Simplicity: Easy to understand and implement, making it an excellent choice for beginners.
- Versatility: Applicable to both classification and regression tasks.
- No Training Period: Doesn’t require an explicit training phase, making it ideal for dynamic datasets.
- Non-parametric: Doesn’t make assumptions about data distribution.
Cons:
- Computational Complexity: Can be slow for large datasets since it needs to calculate distances for every data point.
- Sensitive to Outliers: Outliers can significantly impact the algorithm’s performance.
- Hyperparameter Tuning: Choosing the right value of ‘k’ and distance metric can be challenging.
Comparison with Other Algorithms
k-NN vs. Decision Trees:
- Decision trees build explicit models while k-NN is instance-based.
- Decision trees can handle mixed data types; k-NN typically works with numerical data.
k-NN vs. Naïve Bayes:
- Naïve Bayes is probabilistic, while k-NN is instance-based.
- Naïve Bayes is faster, especially for high-dimensional data.
Example: Classifying Iris Flowers
Let’s walk through a practical example of using k-NN to classify Iris flowers. We’ll create a table to visualize the process step by step.
Sepal Length | Sepal Width | Petal Length | Petal Width | Actual Class | Predicted Class |
---|---|---|---|---|---|
5.1 | 3.5 | 1.4 | 0.2 | Setosa | Setosa |
6.2 | 2.8 | 4.8 | 1.8 | Versicolor | Versicolor |
7.3 | 2.9 | 6.3 | 1.8 | Virginica | Virginica |
Step 1: Choose the value of ‘k.’ In this example, let’s set k = 3.
Step 2: Calculate distances between the new data point and all training data points using a chosen distance metric (e.g., Euclidean).
Step 3: Select the k-nearest neighbors.
Step 4: Determine the majority class among the k-nearest neighbors. In our example, if 2 out of 3 neighbors are Setosa, the predicted class is Setosa.
Step 5: Repeat for all data points.
Real-World Use Cases
The k-NN algorithm finds applications in various fields:
- Medical Diagnosis: Identifying diseases based on patient data.
- Recommendation Systems: Suggesting products or content based on user behavior.
- Anomaly Detection: Detecting fraudulent activities in finance.
- Image and Speech Recognition: Classifying images or transcribing speech.
Conclusion
The k-NN algorithm offers a simple yet effective approach to classification and regression tasks, making it a valuable tool in the machine learning toolbox. Understanding its strengths, weaknesses, and real-world applications can help you harness its power in your data-driven projects. To further explore the k-NN algorithm, consider diving into different distance metrics and evaluation methods for model performance.