- Published on
Exploring Selection Sort: A Simple Sorting Algorithm
- Authors
-
-
- Name
- Jitendra M
- @_JitendraM
-
Table of contents:
-
Introduction
-
How Selection Sort Works
-
Time Complexity of Selection Sort
-
Pseudocode for Selection Sort
-
Python Implementation of Selection Sort
- Pros and Cons
-
Real-World Use Cases
- Comparison with Other Sorting Algorithms
-
Conclusion
-
Explore More Topics
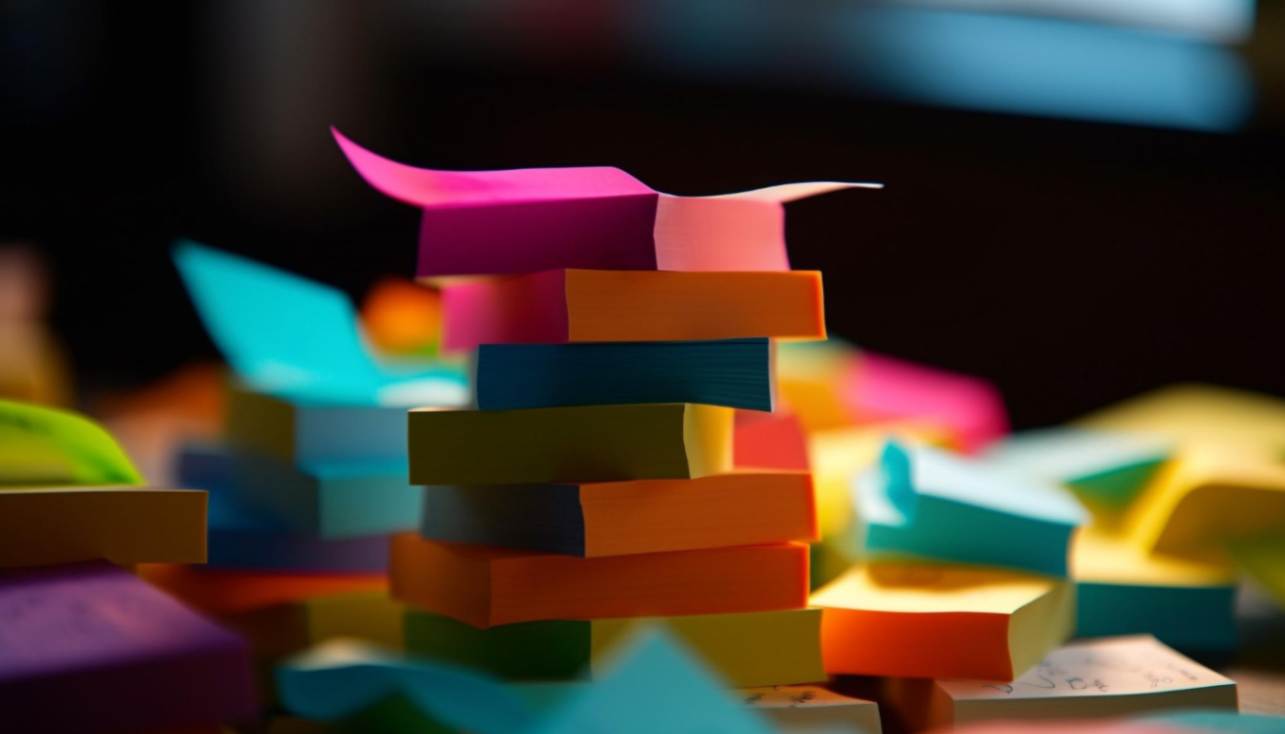
Introduction
Selection Sort is a simple and straightforward sorting algorithm commonly used in computer science. It efficiently sorts a list or array by repeatedly selecting the minimum or maximum element and moving it to its correct position. In this article, we will explore how Selection Sort works, examine its time complexity, discuss its pros and cons, and provide real-world examples of its usage.
How Selection Sort Works
Selection Sort operates by dividing the input list into two parts: the sorted and the unsorted sublists. Initially, the sorted list is empty, while the unsorted list contains all the elements. The algorithm proceeds as follows:
- Find the minimum (or maximum) element in the unsorted sublist.
- Swap the found minimum (or maximum) element with the first element in the unsorted sublist.
- Expand the sorted sublist by one element, and shrink the unsorted sublist by one element.
- Repeat steps 1-3 until the unsorted sublist becomes empty.
The process continues until the entire list is sorted. Selection Sort is known for its simplicity, but it is not the most efficient sorting algorithm for large datasets.
Time Complexity of Selection Sort
Selection Sort has a time complexity of O(n^2), where “n” is the number of elements in the list. This quadratic time complexity makes it inefficient for large datasets. Compared to more advanced sorting algorithms like QuickSort and MergeSort, Selection Sort’s performance degrades significantly as the dataset size increases.
Algorithm | Time Complexity | Efficiency for Large Datasets |
---|---|---|
Selection Sort | O(n^2) | Inefficient |
QuickSort | O(n log n) | Efficient |
MergeSort | O(n log n) | Efficient |
Pseudocode for Selection Sort
Here is the pseudocode for the Selection Sort algorithm:
procedure selectionSort(arr: list)
n = length(arr)
for i = 0 to n-1
minIndex = i
for j = i+1 to n
if arr[j] < arr[minIndex]
minIndex = j
swap arr[i] and arr[minIndex]
The pseudocode provides a step-by-step description of the algorithm’s operation.
Python Implementation of Selection Sort
Let’s implement Selection Sort in Python:
def selection_sort(arr):
n = len(arr)
for i in range(n):
min_index = i
for j in range(i + 1, n):
if arr[j] < arr[min_index]:
min_index = j
arr[i], arr[min_index] = arr[min_index], arr[i]
# Example usage
arr = [64, 34, 25, 12, 22, 11, 90]
selection_sort(arr)
print("Sorted array:", arr)
Pros and Cons
Pros:
- Simplicity: Selection Sort is easy to understand and implement.
- In-Place Sorting: It sorts the array in-place without requiring additional memory.
Cons:
- Inefficiency: Selection Sort has a time complexity of O(n^2), making it inefficient for large datasets.
- Lack of Adaptivity: The algorithm doesn’t adapt to the input data’s existing order.
Real-World Use Cases
While Selection Sort is not commonly used in practice due to its inefficiency, it serves as an educational example of a sorting algorithm. In real-world scenarios, more efficient sorting algorithms like QuickSort or MergeSort are preferred.
Comparison with Other Sorting Algorithms
Selection Sort vs. QuickSort:
- QuickSort is a much faster sorting algorithm and is widely used in practice.
Selection Sort vs. MergeSort:
- MergeSort is a more efficient sorting algorithm and is often preferred for larger datasets.
Conclusion
Selection Sort is a simple sorting algorithm suitable for educational purposes and small datasets. However, it is not efficient for sorting large collections of data, and more advanced sorting algorithms are typically employed in real-world applications.