- Published on
Insertion Sort: A Simple and Efficient Sorting Algorithm Explained
- Authors
-
-
- Name
- Jitendra M
- @_JitendraM
-
Table of contents:
-
Introduction
-
How Insertion Sort Works
-
Time Complexity of Insertion Sort
-
Pseudocode for Insertion Sort
-
Python Implementation of Insertion Sort
-
Optimization Techniques for Insertion Sort
- Pros and Cons
-
Real-World Use Cases
- Comparison with Other Sorting Algorithms
-
Conclusion
-
Explore More Topics
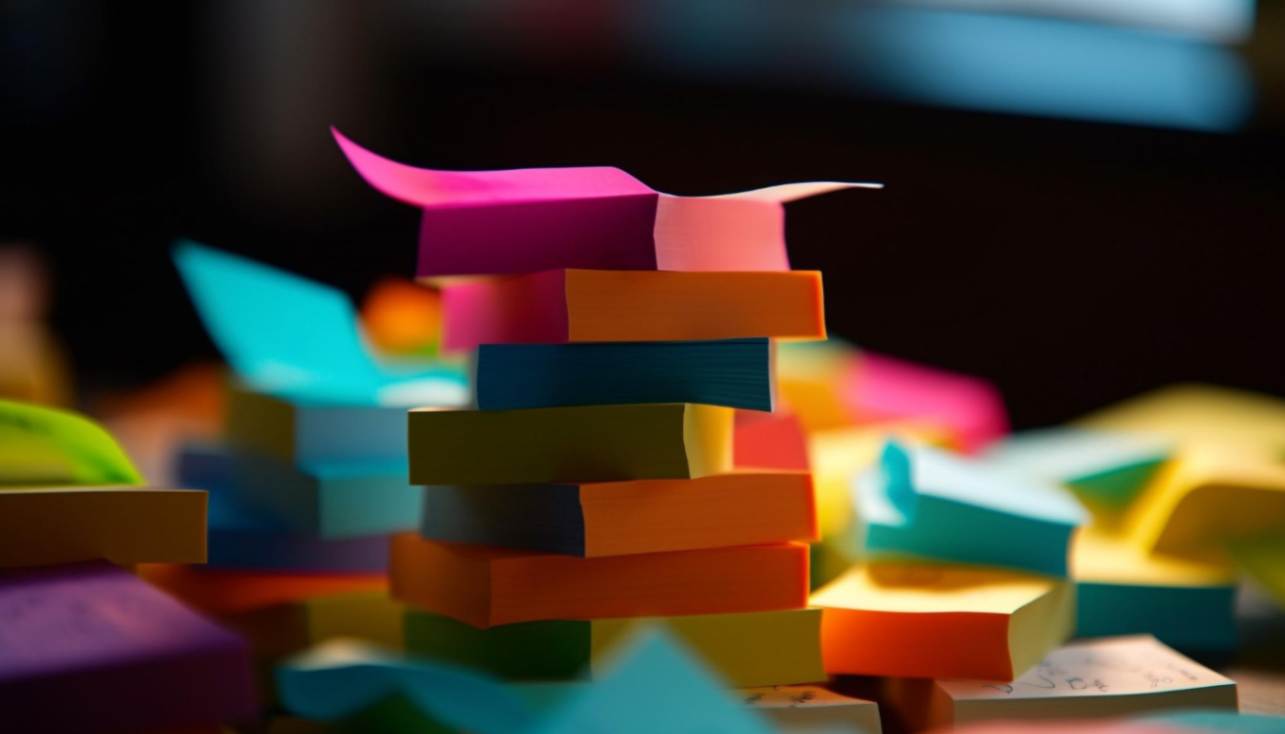
Introduction
Insertion Sort is a simple and efficient sorting algorithm that builds the final sorted array one item at a time. It is particularly useful for small datasets or nearly sorted data. In this article, we will explore how Insertion Sort works, discuss its time complexity, pros, cons, optimization techniques, and provide real-world examples of its usage.
How Insertion Sort Works
Insertion Sort works by iteratively considering one element at a time from the unsorted part of the array and placing it in its correct position within the sorted part of the array. Here’s a step-by-step overview of how Insertion Sort works:
- Start with the second element (index 1) and consider it as the key.
- Compare the key with the previous elements in the sorted part of the array.
- If the key is smaller, shift the previous elements to the right until the correct position for the key is found.
- Insert the key into its correct position.
- Repeat steps 1-4 for each element in the unsorted part of the array.
The process continues until the entire array is sorted. Insertion Sort is known for its simplicity and adaptability to nearly sorted data.
Time Complexity of Insertion Sort
Insertion Sort has an average and worst-case time complexity of O(n^2), making it less efficient than some other sorting algorithms for large datasets. However, it performs well on small datasets and nearly sorted data, where its time complexity approaches O(n).
Sorting Algorithm | Time Complexity (Average Case) | Space Complexity |
---|---|---|
Insertion Sort | O(n^2) | O(1) |
Merge Sort | O(n log n) | O(n) |
Quick Sort | O(n log n) | O(log n) |
Bubble Sort | O(n^2) | O(1) |
Selection Sort | O(n^2) | O(1) |
Heap Sort | O(n log n) | O(1) |
Counting Sort | O(n + k) | O(n + k) |
Radix Sort | O(n * k) | O(n + k) |
Pseudocode for Insertion Sort
Here is the pseudocode for the Insertion Sort algorithm:
InsertionSort(arr):
for i from 1 to length(arr) - 1:
key = arr[i] // Current element to be compared
j = i - 1 // Index of the previous element
// Move elements of arr[0..i-1] that are greater than key
// one position ahead of their current position
while j >= 0 and arr[j] > key:
arr[j + 1] = arr[j] // Shift the larger element to the right
j = j - 1 // Move to the previous element
arr[j + 1] = key // Place the key in its correct position
The pseudocode provides a step-by-step description of the algorithm’s operation.
Python Implementation of Insertion Sort
Let’s implement Insertion Sort in Python:
def insertion_sort(arr):
for i in range(1, len(arr)):
key = arr[i]
j = i - 1
while j >= 0 and key < arr[j]:
arr[j + 1] = arr[j]
j -= 1
arr[j + 1] = key
# Example usage
arr = [12, 11, 13, 5, 6]
insertion_sort(arr)
print("Sorted array:", arr)
In this code snippet, we define an insertion_sort
function that takes an array as input and sorts it in ascending order.
Optimization Techniques for Insertion Sort
Optimization techniques can be applied to improve the performance of Insertion Sort. Some of these techniques include:
-
Binary Insertion Sort: Instead of comparing each element linearly, use binary search to find the correct position for the key, reducing the number of comparisons.
-
Use of Sentinel: Add a sentinel value at the beginning of the array to eliminate the need for bounds checking within the inner loop.
Pros and Cons
Pros:
- Simplicity: Insertion Sort is easy to understand and implement.
- Efficiency for Small Datasets: It performs well on small datasets and nearly sorted data.
- Adaptability: It is adaptive and efficient for partially sorted data.
Cons:
- Inefficiency for Large Datasets: Insertion Sort becomes inefficient for large datasets.
- Quadratic Time Complexity: It has a quadratic time complexity for average and worst cases.
Real-World Use Cases
Insertion Sort, while not the first choice for large-scale data sorting, finds applications in scenarios where simplicity and adaptability matter:
-
Online Retail: Small-scale sorting of customer orders or product listings.
-
Data Entry Systems: Sorting user input data in real-time.
-
Card Games: Sorting a player’s hand in card games.
Comparison with Other Sorting Algorithms
Insertion Sort vs. QuickSort:
- QuickSort is faster for large datasets, but Insertion Sort can be more efficient for small datasets or nearly sorted data.
Insertion Sort vs. Selection Sort:
- Both algorithms have similar time complexities, but Insertion Sort performs better on average due to its adaptive nature.
Conclusion
Insertion Sort is a simple and adaptable sorting algorithm that shines in scenarios with small datasets or nearly sorted data. While it may not be the fastest option for large-scale data, it remains a valuable tool in various real-world applications.