- Published on
Heap Sort: Efficient and Stable Sorting Algorithm
- Authors
-
-
- Name
- Jitendra M
- @_JitendraM
-
Table of contents:
-
Introduction
-
How Heap Sort Works
-
Time Complexity of Heap Sort
-
Space Complexity of Heap Sort
-
Example
-
Pseudocode for Heap Sort
-
Heap Sort in Python
-
Heap Sort in C++
- Pros and Cons of Heap Sort
-
Real-World Use Cases
-
Comparison with Other Sorting Algorithms
-
Conclusion
-
Explore More Topics
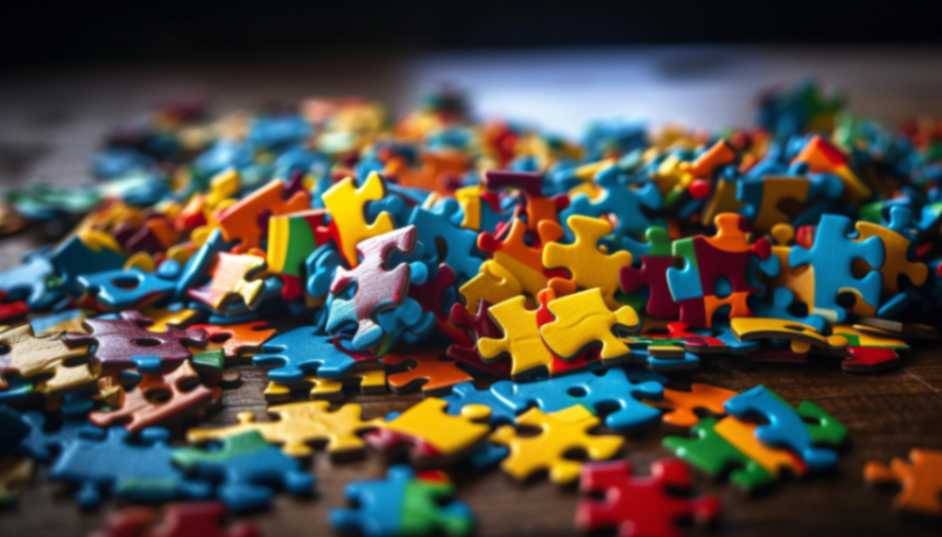
Introduction
Heap Sort is a comparison-based sorting algorithm known for its efficiency and ability to handle large datasets. It falls under the category of “internal sorting,” which means it rearranges data within the main memory of a computer. In this article, we will explore how Heap Sort works, its time complexity, space complexity, pros, cons, real-world applications, and comparisons with other sorting algorithms.
How Heap Sort Works
Heap Sort works by transforming an array into a binary heap—a complete binary tree where each node is greater (or smaller) than its children, depending on whether you’re sorting in ascending or descending order. The basic steps of Heap Sort are as follows:
-
Build a Max Heap: Convert the given array into a max heap, where the root node contains the largest element.
-
Swap and Heapify: Swap the root element (the largest) with the last element in the heap and reduce the size of the heap. Heapify the root node to maintain the max heap property.
-
Repeat: Repeat the swap and heapify process until the entire array is sorted.
-
Result: The array is now sorted in ascending order.
Heap Sort’s ability to convert an array into a max heap and then efficiently sort it makes it a reliable choice for various applications.
Time Complexity of Heap Sort
Heap Sort’s time complexity is as follows:
Time Complexity | Best Case | Average Case | Worst Case |
---|---|---|---|
Heap Sort | O(n log n) | O(n log n) | O(n log n) |
This table format provides a clear overview of Heap Sort’s time complexity in different cases.
Heap Sort’s time complexity is O(n log n)
, which is asymptotically the same as that of Merge Sort. This makes it an efficient sorting algorithm for both small and large datasets.
Space Complexity of Heap Sort
Heap Sort uses in-place sorting, meaning that it sorts the array in memory without using any additional storage. Its space complexity is O(1)
, making it memory-efficient.
Example
Let’s demonstrate Heap Sort with a sample array:
Input: [4, 10, 3, 5, 1]
Step 1: Build a Max Heap:
Heap: [10, 5, 3, 4, 1]
Step 2: Swap and Heapify:
- Swap 10 (root) and 1 (last element).
- Heapify the root.
Heap: [5, 4, 3, 1, 10]
- Swap 5 (root) and 1 (second-to-last element).
- Heapify the root.
Heap: [4, 1, 3, 5, 10]
Step 3: Repeat until sorted:
- Swap 4 (root) and 1 (last element).
- Heapify the root.
Heap: [3, 1, 4, 5, 10]
- Swap 3 (root) and 1 (second-to-last element).
- Heapify the root.
Heap: [1, 3, 4, 5, 10]
Result: The array is now sorted in ascending order. [1, 3, 4, 5, 10]
Pseudocode for Heap Sort
Here’s a simplified pseudocode for Heap Sort:
function heapSort(arr):
buildMaxHeap(arr)
for i from n-1 to 0:
swap arr[0] and arr[i]
heapify(arr, i, 0)`
Heap Sort in Python
Here’s a Python program for Heap Sort:
def heapify(arr, n, i):
largest = i
left = 2 * i + 1
right = 2 * i + 2
if left < n and arr[i] < arr[left]:
largest = left
if right < n and arr[largest] < arr[right]:
largest = right
if largest != i:
# Swap elements
arr[i], arr[largest] = arr[largest], arr[i]
heapify(arr, n, largest)
def heapSort(arr):
n = len(arr)
# Build a max heap
for i in range(n // 2 - 1, -1, -1):
heapify(arr, n, i)
# Extract elements one by one
for i in range(n - 1, 0, -1):
# Swap elements
arr[i], arr[0] = arr[0], arr[i]
heapify(arr, i, 0)
# Example uses
arr = [12, 11, 13, 5, 6, 7]
print("Original array:", arr)
heapSort(arr)
print("Sorted array:", arr)
Heap Sort in C++
Here’s a C++ program for Heap Sort:
#include <iostream>
using namespace std;
void heapify(int arr[], int n, int i) {
int largest = i;
int left = 2 * i + 1;
int right = 2 * i + 2;
if (left < n && arr[i] < arr[left])
largest = left;
if (right < n && arr[largest] < arr[right])
largest = right;
if (largest != i) {
// Swap elements
swap(arr[i], arr[largest]);
heapify(arr, n, largest);
}
}
void heapSort(int arr[], int n) {
for (int i = n / 2 - 1; i >= 0; i--)
heapify(arr, n, i);
for (int i = n - 1; i >= 0; i--) {
// Swap elements
swap(arr[0], arr[i]);
heapify(arr, i, 0);
}
}
int main() {
int arr[] = {4, 10, 3, 5, 1};
int n = sizeof(arr) / sizeof(arr[0]);
heapSort(arr, n);
cout << "Sorted array: ";
for (int i = 0; i < n; i++)
cout << arr[i] << " ";
cout << endl;
return 0;
}
This concludes our exploration of Heap Sort, including its example, pseudocode, and implementations in Python and C++. Heap Sort’s efficiency and stability make it a valuable sorting algorithm for various applications.
Pros and Cons of Heap Sort
Pros:
-
Efficiency: Heap Sort is highly efficient and performs consistently well for large datasets.
-
In-Place Sorting: It sorts the array in-place, minimizing memory usage.
-
Stability: Heap Sort is a stable sorting algorithm, meaning it preserves the relative order of equal elements.
Cons:
-
Not as Fast as Quick Sort: While efficient, Heap Sort may not be as fast as Quick Sort for small and medium-sized datasets.
-
Not as Widely Used: Heap Sort is not as commonly used as some other sorting algorithms in certain programming languages and libraries.
Real-World Use Cases
Heap Sort is used in various real-world applications, including:
-
Operating Systems: Heap Sort is used by operating systems during memory allocation and resource management.
-
Priority Queues: It is employed in data structures like priority queues to maintain the order of elements efficiently. Priority queues are used in scenarios like task scheduling and network routing.
-
External Sorting: Heap Sort is used in external sorting algorithms when dealing with large datasets that don’t fit entirely in memory.
Heap Sort’s efficiency and stability make it a valuable sorting algorithm for various applications, especially when memory usage is a concern.
Comparison with Other Sorting Algorithms
Heap Sort offers a predictable and efficient sorting solution. Here’s a brief comparison with other sorting algorithms, including Quick Sort and Merge Sort:
Characteristic | Heap Sort | Quick Sort | Merge Sort |
---|---|---|---|
Efficiency | Efficient | Comparable to Quick Sort | Efficient |
Stability | Stable | Not stable | Stable |
Space Complexity | O(1) | O(log n) | O(n) |
Suitable for Large Datasets | Yes | Yes | Yes |
-
Efficiency: Heap Sort is efficient and, in terms of time complexity, is comparable to Quick Sort for most cases. However, Quick Sort may outperform Heap Sort for small and medium-sized datasets due to its lower overhead.
-
Stability: Heap Sort is a stable sorting algorithm, unlike Quick Sort.
-
Space Complexity: Heap Sort excels in space efficiency, as it uses in-place sorting with constant additional memory.
When selecting a sorting algorithm, consider your specific requirements and constraints.
Conclusion
Heap Sort is a reliable sorting algorithm known for its efficiency and stability. Its ability to handle large datasets and use minimal memory resources makes it a valuable choice in various applications. By converting an array into a max heap and efficiently sorting it, Heap Sort provides a predictable and efficient sorting solution. In summary, Heap Sort is a versatile and efficient sorting algorithm suitable for a wide range of scenarios, especially when memory efficiency is a concern.