- Published on
Quick Sort: A Powerful Sorting Algorithm
- Authors
-
-
- Name
- Jitendra M
- @_JitendraM
-
Table of contents:
-
Introduction
-
How Quick Sort Works
-
Pseudocode for Quick Sort
-
Quick Sort in Python
-
Quick Sort in C++
-
Time Complexity of Quick Sort
-
Space Complexity of Quick Sort
- Pros and Cons of Quick Sort
-
Real-World Use Cases
-
Limitations of Quick Sort
-
Comparison with Other Sorting Algorithms
-
Conclusion
-
Explore More Topics
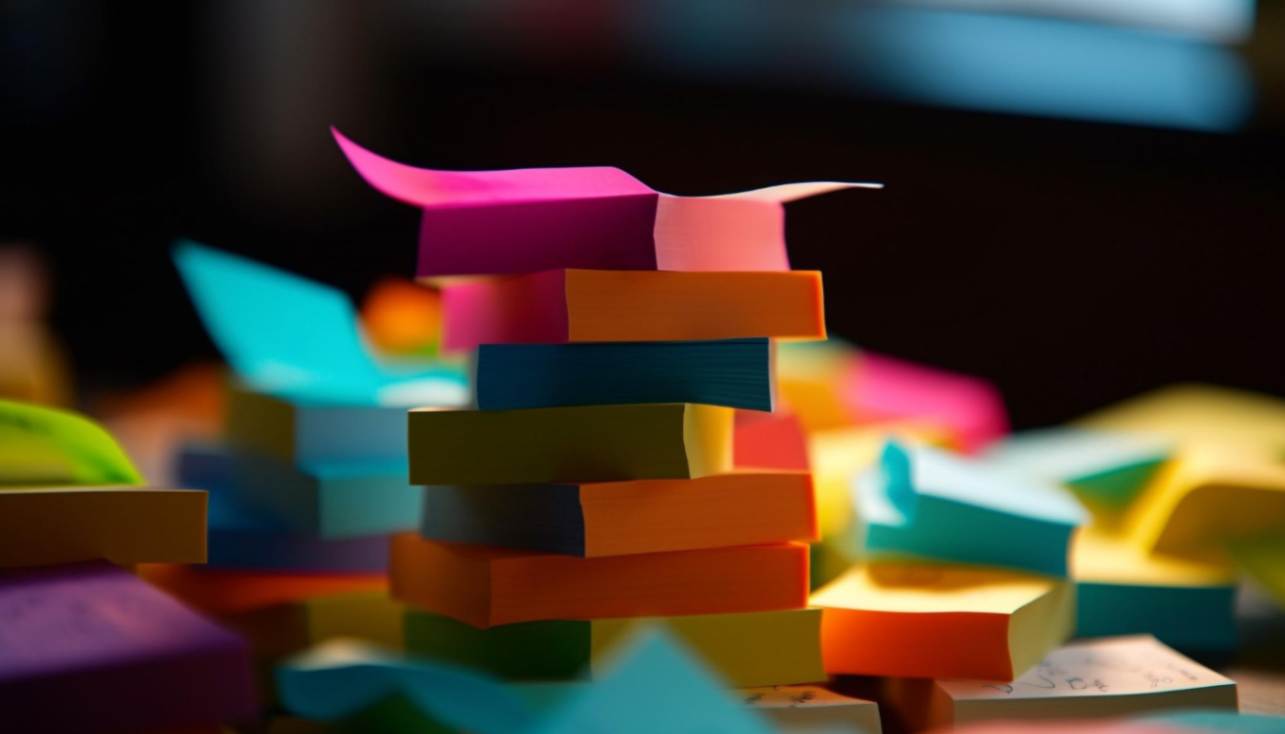
Introduction
Quick Sort is a highly efficient and widely used sorting algorithm known for its speed and effectiveness. It’s a divide-and-conquer algorithm that sorts an array or list by selecting a “pivot” element and partitioning the other elements into two sub-arrays, according to whether they are less than or greater than the pivot. In this article, we will explore how Quick Sort works, its time complexity, space complexity, pros, cons, real-world applications, and limitations.
How Quick Sort Works
Quick Sort’s efficiency lies in its divide-and-conquer strategy:
-
Choose a Pivot: Select a pivot element from the array.
-
Partitioning: Reorder the array so that all elements less than the pivot come before all elements greater than the pivot. The pivot is now in its final sorted position.
-
Recursion: Recursively sort the sub-arrays of elements less than and greater than the pivot.
-
Combine: Combine the sorted sub-arrays with the pivot to get the final sorted array.
This process continues until the entire array is sorted. Quick Sort is known for its speed and ability to handle large datasets efficiently.
Pseudocode for Quick Sort
Here’s a pseudocode representation of the Quick Sort algorithm:
procedure quickSort(arr: array)
if length(arr) ≤ 1
return arr
pivot = selectPivot(arr) # Choose a pivot element
left = [] # Elements less than pivot
right = [] # Elements greater than pivot
equal = [] # Elements equal to pivot
for each element in arr
if element < pivot
append element to left
else if element > pivot
append element to right
else
append element to equal
left = quickSort(left) # Recursively sort left sub-array
right = quickSort(right) # Recursively sort right sub-array
return concatenate(left, equal, right) # Combine sorted sub-arrays
The pseudocode outlines the key steps of the Quick Sort algorithm.
Quick Sort in Python
Here’s a Python implementation of Quick Sort:
def quick_sort(arr):
if len(arr) <= 1:
return arr
pivot = arr[len(arr) // 2] # Choose a pivot element
left = [x for x in arr if x < pivot]
equal = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quick_sort(left) + equal + quick_sort(right)
# Example usage:
arr = [12, 4, 5, 6, 7, 3, 1, 15]
sorted_arr = quick_sort(arr)
print("Sorted array:", sorted_arr)
Quick Sort in C++
Here’s a C++ implementation of Quick Sort:
#include <iostream>
#include <vector>
using namespace std;
int partition(vector<int> &arr, int low, int high) {
int pivot = arr[high]; // Choose the rightmost element as the pivot
int i = (low - 1); // Index of smaller element
for (int j = low; j <= high - 1; j++) {
if (arr[j] < pivot) {
i++;
swap(arr[i], arr[j]);
}
}
swap(arr[i + 1], arr[high]);
return (i + 1);
}
void quick_sort(vector<int> &arr, int low, int high) {
if (low < high) {
int pi = partition(arr, low, high);
quick_sort(arr, low, pi - 1);
quick_sort(arr, pi + 1, high);
}
}
int main() {
vector<int> arr = {12, 4, 5, 6, 7, 3, 1, 15};
int n = arr.size();
cout << "Unsorted array: ";
for (int i = 0; i < n; i++) {
cout << arr[i] << " ";
}
quick_sort(arr, 0, n - 1);
cout << "\nSorted array: ";
for (int i = 0; i < n; i++) {
cout << arr[i] << " ";
}
return 0;
}
Time Complexity of Quick Sort
Quick Sort’s time complexity is as follows:
Case | Time Complexity |
---|---|
Best Case | O(n log n) |
Average Case | O(n log n) |
Worst Case | O(n^2) |
The worst-case time complexity occurs when the pivot selection consistently results in unbalanced partitions. However, efficient pivot selection strategies, like the median-of-three, minimize the likelihood of the worst case.
Space Complexity of Quick Sort
Quick Sort has an average space complexity of O(log n)
due to the recursive call stack. However, in the worst case, it can have a space complexity of O(n)
when unbalanced partitions are consistently chosen.
Pros and Cons of Quick Sort
Pros:
-
Efficiency: Quick Sort is one of the fastest sorting algorithms for most datasets.
-
In-Place Sorting: It sorts the array in-place, minimizing memory usage.
-
Average-Case Performance: Quick Sort’s average-case time complexity of O(n log n) is highly efficient.
Cons:
-
Worst-Case Performance: In the worst case, when unbalanced partitions occur consistently, the time complexity degrades to O(n^2).
-
Not Stable: Quick Sort is not a stable sorting algorithm, meaning it may change the relative order of equal elements.
Real-World Use Cases
Quick Sort is widely used in real-world applications, including:
-
General Sorting: Sorting large datasets efficiently in various software applications.
-
Programming Languages: Many programming languages and libraries use Quick Sort as their default sorting algorithm, including Python and Java.
-
Database Management: Sorting data in databases to improve query performance.
Limitations of Quick Sort
While Quick Sort is a powerful sorting algorithm, it has its limitations:
-
Instability: Quick Sort is not stable, meaning it may change the relative order of equal elements.
-
Worst-Case Scenario: In the worst-case scenario, when pivot selection consistently results in unbalanced partitions, Quick Sort’s performance can degrade to
O(n^2)
.
Quick Sort’s efficiency, in-place sorting, and average-case performance make it a valuable tool for sorting large datasets quickly and effectively. However, careful consideration is needed for worst-case scenarios and stability requirements.
Comparison with Other Sorting Algorithms
Quick Sort is a highly efficient sorting algorithm, but how does it compare to other commonly used sorting algorithms? Let’s take a closer look at their characteristics:
Characteristic | Quick Sort | Merge Sort | Heap Sort | Insertion Sort |
---|---|---|---|---|
Efficiency | Highly efficient for most datasets | Highly efficient for most datasets | Moderate to slow for small datasets | Poor for large datasets |
Stability | Not stable | Stable | Not stable | Not stable |
Space Complexity | O(log n) avg. | O(n) | O(1) | O(1) avg. |
O(n) worst | O(n^2) worst | |||
Suitable for Large Datasets | Yes | Yes | Yes | No |
In-Place Sorting | Yes | No | Yes | Yes |
-
Efficiency: Quick Sort is highly efficient for most datasets. Merge Sort is also highly efficient but more stable. Heap Sort is moderate to slow for small datasets. Insertion Sort is poor for large datasets.
-
Stability: Merge Sort is the only stable sorting algorithm among these options.
-
Space Complexity: Quick Sort has an average space complexity of O(log n), while Merge Sort has O(n) space complexity. Heap Sort is in-place with O(1) space complexity, and Insertion Sort has O(1) average space complexity but O(n^2) worst-case space complexity.
-
Suitable for Large Datasets: Quick Sort and Merge Sort are suitable for large datasets. Heap Sort is efficient for all dataset sizes. Insertion Sort is not recommended for large datasets.
-
In-Place Sorting: Quick Sort, Heap Sort, and Insertion Sort are in-place sorting algorithms, while Merge Sort requires additional memory.
When choosing a sorting algorithm, consider the specific requirements of your application, dataset size, and the stability of the algorithm. Quick Sort is an excellent choice for many scenarios, but the best algorithm depends on your use case.
Conclusion
In summary, Quick Sort is a versatile and highly efficient sorting algorithm with applications in a wide range of fields. Its speed, efficiency, and in-place sorting make it a valuable tool for sorting large datasets quickly and effectively.